Campaigns Endpoints
View and manage all your campaigns
A FEW THINGS TO KNOW
Learn more about campaign management with our API Here!
Endpoints
Verb | Endpoint | Description |
---|---|---|
POST | /accounts/{accountId}/campaigns | Create a Campaign |
GET | /accounts/{accountId}/campaigns | Get All Campaigns |
GET | /campaigns/{campaignId} | Get a Specific Campaign |
PUT | /campaigns/{campaignId} | Update a Specific Campaign |
Info
- Create operations using the
POST
method expect every Required field; omitting Optional fields will set those fields to Default values- Update operations using the
PUT
method except for every Write field; omitting these fields is equivalent to setting them tonull
, if possible
Campaign Attributes
Data Type: string
Description: Campaign ID
Accepted Value: int64
Default Values: None
Write? No
Nullable? No
accountId Required
Data Type: string
Description: Account ID
Accepted Value: int64
Default Value: None
Write? No
Nullable? No
name Required
Data Type: string
Description: Campaign name; must be unique within an account
Values: 255 char limit
Default Value: None
Write? Yes
Nullable? No
type Optional
Data Type: enum
Description: Campaing type
Accepted Values: auction
,preferred
Default Value: auction
Write? None other than the accepted values
Nullable? No - if attribute is passed in the call, a value must be specified
budget Optional
Data Type: number
Description: Campaign lifetime spend cap; uncapped if omitted or set to null.
- Optional when campaignType = auction
- Mandatory when campaignType = preferred
Accepted Values: at least 0
Default Value: null
Write? Yes
Nullable? Yes
budgetSpent Optional
Data Type: number
Description: Amount the campaign has already spent
Accepted Values: at least 0
Default Value: 0.0
Write? No
Nullable? No
budgetRemaining
Data Type: number
Description: Amount the campaign has remaining until cap is hit; null
if budget is uncapped
Accepted Values: between 0 and budget
Amount
Default Value: null
Write? No
Nullable? Yes
clickAttributionWindow Required
Data Type: enum
Description: Post-click attribution window
Accepted Values: 7D
,14D
, 30D
Default Value: 30D
Write? Yes
Nullable? No
viewAttributionWindow Required
Data Type: enum
Description: Post-click attribution scope
Accepted Values: samesku
, sameskucategory
, sameskucategorybrand
Default Value: sameskucategory
Write? None other than the accepted values
Nullable? No
viewAttributionScope
Data Type: enum
Description: Post-view attribution scope
Accepted Values: samesku
, sameskucategory
, sameskucategorybrand
Default Value: sameskucategory
Write? None other than the accepted values
Nullable? No
promotedBrandIds
Data Type: string array
Description: Set of brands represented in line items of the campaign; note this set is append only
Accepted Values: list of brandId
Default Value: [ ]
Write? No
Nullable? No
drawableBalanceIds
Data Type: string array
Description: List of balances the campaign is able to draw from; at least one balance is required for a campaign to begin
Accepted Values: list of balanceId
Default Value: [ ]
Write? No
Nullable? No
status
Data Type: enum
Description: Campaign status, derived from the status of line items it holds; active if at least one line item is active. To understand the conditions that will cause a status to change, check out our status page
Accepted Values: active
, inactive
Default Value: inactive
Write? No
Nullable? No
createdAt
Data Type: timestamp
Description: Timestamp in UTC of campaign creation
Accepted Values: -ISO-8601
Default Value: None
Write? No
Nullable? No
createdAt
Data Type: timestamp
Description: Timestamp in UTC of last campaign update
Accepted Values: ISO-8601
Default Value: None
Write? No
Nullable? No
Create a Campaign
This endpoint creates a new campaign in the specified account.
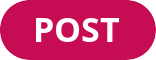
https://api.criteo.com/2022-07/retail-media/accounts/{accountId}/campaigns
Sample Request
curl -X POST "https://api.criteo.com/2022-07/retail-media/accounts/18446744073709551616/campaigns" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>" \
-H "Content-Type: application/json" \
-d '{
"data": {
"type": "RetailMediaCampaign",
"attributes": {
"name": "My New Campaign"
}
}
}'
import requests
import json
url = "https://api.criteo.com/2022-07/retail-media/accounts/4/campaigns"
payload = json.dumps({
"data": {
"type": "<string>",
"attributes": {
"name": "New API Campaign",
"type": "auction",
"clickAttributionWindow": "30D",
"budget": "2.00",
"clickAttributionScope": "samesku"
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"data\": {\n \"type\": \"<string>\",\n \"attributes\": {\n \"name\": \"New API Campaign\",\n \"type\": \"auction\",\n \"clickAttributionWindow\": \"30D\",\n \"budget\": \"2.00\",\n \"clickAttributionScope\": \"samesku\"\n\n }\n }\n}");
Request request = new Request.Builder()
.url("https://api.criteo.com/2022-07/retail-media/accounts/4/campaigns")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2022-07/retail-media/accounts/4/campaigns');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{\n "data": {\n "type": "<string>",\n "attributes": {\n "name": "New API Campaign",\n "type": "auction",\n "clickAttributionWindow": "30D",\n "budget": "2.00",\n "clickAttributionScope": "samesku"\n\n }\n }\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaCampaign",
"id": "3683145960016759663",
"attributes": {
"accountId": "18446744073709551616",
"name": "My New Campaign",
"type": "auction",
"promotedBrandIds": [],
"drawableBalanceIds": [],
"budget": null,
"budgetSpent": 0.00,
"budgetRemaining": null,
"clickAttributionWindow": "30D",
"viewAttributionWindow": "1D",
"clickAttributionScope": "sameSkuCategory",
"viewAttributionScope": "sameSkuCategory",
"status": "inactive",
"createdAt": "2020-06-03T14:17:57+00:00",
"updatedAt": "2020-06-03T14:17:57+00:00"
}
}
}
Get All Campaigns
This endpoint lists all campaigns in the specified account. Results are paginated.
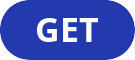
https://api.criteo.com/2022-07/retail-media/accounts/{accountId}/campaigns
Sample Request
curl -X GET "https://api.criteo.com/2022-07/retail-media/accounts/18446744073709551616/campaigns" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
import requests
url = "https://api.criteo.com/2022-07/retail-media/accounts/4/campaigns"
payload={}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/2022-07/retail-media/accounts/4/campaigns")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2022-07/retail-media/accounts/4/campaigns');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"type": "RetailMediaCampaign",
"id": "8343086999167541140",
"attributes": {
"accountId": "18446744073709551616",
"name": "Campaign 123",
"type": "auction",
"promotedBrandIds": ["7672171780147256541",
"5979998329674492121"],
"drawableBalanceIds": ["14094543095747588032"],
"budget": 100000.00,
"budgetSpent": 6172.33,
"budgetRemaining": 93827.67,
"clickAttributionWindow": "30D",
"viewAttributionWindow": "none",
"clickAttributionScope": "sameSkuCategory",
"viewAttributionScope": "sameSkuCategory",
"status": "active",
"createdAt": "2020-04-06T13:01:07+00:00",
"updatedAt": "2020-06-03T11:37:53+00:00"
}
},
// ...
{
"type": "RetailMediaCampaign",
"id": "16108177282234788969",
"attributes": {
"accountId": "18446744073709551616",
"name": "Campaign 789",
"type": "auction",
"promotedBrandIds": ["5979998329674492121"],
"drawableBalanceIds": [],
"budget": null,
"budgetSpent": 0.00,
"budgetRemaining": null,
"clickAttributionWindow": "7D",
"viewAttributionWindow": "1D",
"clickAttributionScope": "sameSkuCategory",
"viewAttributionScope": "sameSkuCategory",
"status": "inactive",
"createdAt": "2020-04-06T23:47:11+00:00",
"updatedAt": "2020-04-06T23:47:11+00:00"
}
}
],
"metadata": {
"totalItemsAcrossAllPages": 95,
"currentPageSize": 25,
"currentPageIndex": 0,
"totalPages": 4,
"nextPage": "https://api.criteo.com/2022-07/retail-media/accounts/18446744073709551616/campaigns?pageIndex=1&pageSize=25",
"previousPage": null
}
}
Get a Specific Campaign
This endpoint retrieves the specified campaign.
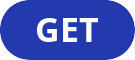
https://api.criteo.com/2022-07/retail-media/campaigns/{campaignId}
Sample Request
curl -X GET "https://api.criteo.com/2022-04/retail-media/campaigns/8343086999167541140" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
import requests
url = "https://api.criteo.com/2022-07/retail-media/campaigns/1280"
payload={}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/2022-07/retail-media/campaigns/1280")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2022-07/retail-media/campaigns/1280');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaCampaign",
"id": "8343086999167541140",
"attributes": {
"accountId": "18446744073709551616",
"name": "Campaign 123",
"type": "auction",
"promotedBrandIds": ["7672171780147256541",
"5979998329674492121"],
"drawableBalanceIds": ["14094543095747588032"],
"budget": 100000.00,
"budgetSpent": 6172.33,
"budgetRemaining": 93827.67,
"clickAttributionWindow": "30D",
"viewAttributionWindow": "none",
"clickAttributionScope": "sameSkuCategory",
"viewAttributionScope": "sameSkuCategory",
"status": "active",
"createdAt": "2020-04-06T13:01:07+00:00",
"updatedAt": "2020-06-03T11:37:53+00:00"
}
}
}
Update a Specific Campaign
This endpoint updates the specified campaign. In this example, we switch to an uncapped campaign budget and update the post-view attribution window.
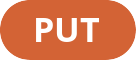
https://api.criteo.com/2022-07/retail-media/campaigns/{campaignId}
Sample Request
curl -X PUT "https://api.criteo.com/2022-07/retail-media/campaigns/8343086999167541140" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>" \
-H "Content-Type: application/json" \
-d '{
"data": {
"type": "RetailMediaCampaign",
"id": "8343086999167541140",
"attributes": {
"name": "Campaign 123",
"budget": null,
"clickAttributionWindow": "30D",
"viewAttributionWindow": "1D",
"clickAttributionScope": "sameskucategory",
"viewAttributionScope": "sameskucategory"
}
}
}'
import requests
import json
url = "https://api.criteo.com/2022-07/retail-media/campaigns/76216196459831296"
payload = json.dumps({
"data": {
"type": "RetailMediaCampaign",
"id": "76216196459831296",
"attributes": {
"name": "api campaign",
"budget": None,
"clickAttributionWindow": "30D",
"viewAttributionWindow": "1D",
"clickAttributionScope": "sameskucategory",
"viewAttributionScope": "sameskucategory"
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("PUT", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"data\": {\n \"type\": \"RetailMediaCampaign\",\n \"id\": \"76216196459831296\",\n \"attributes\": {\n \"name\": \"api campaign\",\n \"budget\": null,\n \"clickAttributionWindow\": \"30D\",\n \"viewAttributionWindow\": \"1D\",\n \"clickAttributionScope\": \"sameskucategory\",\n \"viewAttributionScope\": \"sameskucategory\"\n }\n }\n }");
Request request = new Request.Builder()
.url("https://api.criteo.com/2022-07/retail-media/campaigns/76216196459831296")
.method("PUT", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2022-07/retail-media/campaigns/76216196459831296');
$request->setMethod(HTTP_Request2::METHOD_PUT);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{\n "data": {\n "type": "RetailMediaCampaign",\n "id": "76216196459831296",\n "attributes": {\n "name": "api campaign",\n "budget": null,\n "clickAttributionWindow": "30D",\n "viewAttributionWindow": "1D",\n "clickAttributionScope": "sameskucategory",\n "viewAttributionScope": "sameskucategory"\n }\n }\n }');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaCampaign",
"id": "8343086999167541140",
"attributes": {
"accountId": "18446744073709551616",
"name": "Campaign 123",
"type": "auction",
"promotedBrandIds": ["7672171780147256541",
"5979998329674492121"],
"drawableBalanceIds": ["14094543095747588032"],
"budget": null,
"budgetSpent": 6172.33,
"budgetRemaining": null,
"clickAttributionWindow": "30D",
"viewAttributionWindow": "1D",
"clickAttributionScope": "sameSkuCategory",
"viewAttributionScope": "sameSkuCategory",
"status": "active",
"createdAt": "2020-04-06T13:01:07+00:00",
"updatedAt": "2020-06-03T14:23:28+00:00"
}
}
}
Responses
Response | Description |
---|---|
![]() 200 | Call completed with success |
![]() 201 | Campaign was created successfully |
![]() 400 | Validation Error - one ore more required field was not found. Confirm if all required fields are present in the API call |
Updated over 1 year ago