Fill Rate Report
The fill rate report measures the impressions that are successfully filled by ads in comparison to the total number of available impressions. Retailers can leverage this data to analyze areas in demand and their supply that are impacting the ability to maximize yield.
Endpoints
The report generation uses an asynchronous endpoint that is used to receive the report creation request (using a POST request); then, using the report id
generated, it's possible to check the report status and download the output results using the following GET endpoint requests.
Verb | Endpoint | Description |
---|---|---|
POST | /reports/fillrate | Request a fill rate report creation |
GET | /reports/{reportId}/status | Get status of a specific report |
GET | /reports/{reportId}/output | Download output of a specific report |
Report Request Attributes
Attribute | Data Type | Description |
---|---|---|
supplyAccountIds * | list | Supply Account IDs to pull results for Accepted values: array of strings/int64 Writeable? N / Nullable? N |
dimensions * | list | An array of strings used to define which dimensions to see in the report Accepted values: refer to Metrics and Dimensions for the complete list of supported dimensions Writeable? N / Nullable? N |
metrics * | list | An array of strings used to define which metrics to see in the report Accepted values: refer to Metrics and Dimensions for the complete list of supported metrics Writeable? N / Nullable? N |
startDate * | date | Start date of the report (inclusive) Accepted values: yyyy-mm-dd with max interval of 100 days with endDate Writeable? N / Nullable? N |
endDate * | date | End date of the report (inclusive) Accepted values: yyyy-mm-dd with max interval of 100 days with startDate Writeable? N / Nullable? N |
timezone | string | Time zone to consider in the metrics calculation,startDate and endDate Accepted values: IANA (TZ database) time zones (example: America/New_York , Europe/Paris , Asia/Tokyo , UTC )Default: UTC Writeable? N / Nullable? Y |
format | enum | The format type the report should return results Accepted values: json , json-compact , json-newline , csv Default: json Writeable? N / Nullable? N |
* Required
Metrics and Dimensions
For a complete list of all supported metrics and dimension, check out the Metrics and Dimensions
Reporting Asynchronous Workflow: Step 1 of 3
- First, create a request for the supply account report with the desired attributes
- This generates a
reportId
representing the report
Create a Fill Rate Report request
This endpoint receives requests to create Fill Rate Reports and returns a report id
(to be used in the next steps) in case the request was successfully created ; otherwise, will expose errors details about the request issues
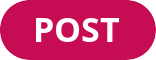
https://api.criteo.com/preview/retail-media/reports/fillrate
Sample Request
curl -L -X POST 'https://api.criteo.com/preview/retail-media/reports/fillrate' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": {
"type": "FillRateReportingAPI",
"attributes": {
"supplyAccountIds": [
"168787661xxxxxxxxx",
"164684223xxxxxxxxx"
],
"startDate": "2025-01-01",
"endDate": "2025-01-31",
"dimensions": [
"date",
"retailerId",
"retailerName",
"placementId",
"placementName",
"pageTypeName",
"environment",
"servedCategory",
"retailerCategoryId",
"retailerCategoryName"
],
"metrics": [
"pageViews",
"availablePlacements",
"unfilledPlacements",
"fillRate",
"placementImpressions",
"productImpressions",
"impressions",
"placementClicks",
"productClicks",
"clicks",
"placementImpressionsCTR",
"productImpressionsCTR",
"cpm",
"cpc",
"placementImpressionsRevenue",
"productClicksRevenue",
"totalRevenue",
"workingMedia",
"netRevenue",
"uniqueVisitors",
"frequency"
],
"format": "csv",
"timezone": "America/New_York"
}
}
}'
import requests
import json
url = "https://api.criteo.com/preview/retail-media/reports/fillrate"
payload = json.dumps({
"data": {
"type": "FillRateReportingAPI",
"attributes": {
"supplyAccountIds": [
"168787661166874264",
"164684223544883213"
],
"startDate": "2025-01-01",
"endDate": "2025-01-31",
"dimensions": [
"date",
"retailerId",
"retailerName",
"placementId",
"placementName",
"pageTypeName",
"environment",
"servedCategory",
"retailerCategoryId",
"retailerCategoryName"
],
"metrics": [
"pageViews",
"availablePlacements",
"unfilledPlacements",
"fillRate",
"placementImpressions",
"productImpressions",
"impressions",
"placementClicks",
"productClicks",
"clicks",
"placementImpressionsCTR",
"productImpressionsCTR",
"cpm",
"cpc",
"placementImpressionsRevenue",
"productClicksRevenue",
"totalRevenue",
"workingMedia",
"netRevenue",
"uniqueVisitors",
"frequency"
],
"format": "csv",
"timezone": "America/New_York"
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"data\":{\"type\":\"FillRateReportingAPI\",\"attributes\":{\"supplyAccountIds\":[\"168787661166874264\",\"164684223544883213\"],\"startDate\":\"2025-01-01\",\"endDate\":\"2025-01-31\",\"dimensions\":[\"date\",\"retailerId\",\"retailerName\",\"placementId\",\"placementName\",\"pageTypeName\",\"environment\",\"servedCategory\",\"retailerCategoryId\",\"retailerCategoryName\"],\"metrics\":[\"pageViews\",\"availablePlacements\",\"unfilledPlacements\",\"fillRate\",\"placementImpressions\",\"productImpressions\",\"impressions\",\"placementClicks\",\"productClicks\",\"clicks\",\"placementImpressionsCTR\",\"productImpressionsCTR\",\"cpm\",\"cpc\",\"placementImpressionsRevenue\",\"productClicksRevenue\",\"totalRevenue\",\"workingMedia\",\"netRevenue\",\"uniqueVisitors\",\"frequency\"],\"format\":\"csv\",\"timezone\":\"America/New_York\"}}}");
Request request = new Request.Builder()
.url("https://api.criteo.com/preview/retail-media/reports/fillrate")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/preview/retail-media/reports/fillrate');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{"data":{"type":"FillRateReportingAPI","attributes":{"supplyAccountIds":["168787661166874264","164684223544883213"],"startDate":"2025-01-01","endDate":"2025-01-31","dimensions":["date","retailerId","retailerName","placementId","placementName","pageTypeName","environment","servedCategory","retailerCategoryId","retailerCategoryName"],"metrics":["pageViews","availablePlacements","unfilledPlacements","fillRate","placementImpressions","productImpressions","impressions","placementClicks","productClicks","clicks","placementImpressionsCTR","productImpressionsCTR","cpm","cpc","placementImpressionsRevenue","productClicksRevenue","totalRevenue","workingMedia","netRevenue","uniqueVisitors","frequency"],"format":"csv","timezone":"America/New_York"}}}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response: Report request successfully created (response status 🟢 200
)
{
"data": {
"id": "5f148e12-fba3-432e-b0d5-fe316xxxxxxx",
"type": "StatusResponse",
"attributes": {
"id": "5f148e12-fba3-432e-b0d5-fe3xxxxxx",
"status": "pending",
"rowCount": 0,
"fileSizeBytes": 0,
"md5CheckSum": null,
"createdAt": "2025-02-14T14:59:20.281Z",
"expiresAt": null,
"message": null
}
},
"warnings": [],
"errors": []
}
Reporting Asynchronous Workflow: Step 2 of 3
- Next, use the
reportId
to pull the report status endpoint until one is successfully computed
Sample Response: Validation error when creating report request (response status 🔴 400
)
{
"warnings": [],
"errors": [
{
"traceId": "1cb17c306c732b47a4f29aa4xxxxxx",
"traceIdentifier": "1cb17c306c732b47a4f29axxxxxx",
"type": "validation",
"code": "deserialization-error",
"instance": "/reports/async/fillrate",
"title": "Deserialization error",
"detail": "Json deserialization failed : Error converting value \"xls\". Path 'data.attributes.format', line 46, position 27."
}
]
}
Get status of specific report
This endpoint retrieves the status of a specific report creation. Status can be pending
, success
, failure
, or expired
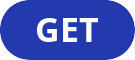
https://api.criteo.com/preview/retail-media/reports/{reportId}/status
Sample Request
curl -L 'https://api.criteo.com/preview/retail-media/reports/5f148e12-fba3-432e-b0d5-fe316xxxxx/status' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>'
Sample Response
{
"data": {
"id": "5f148e12-fba3-432e-b0d5-fe316xxxxxx",
"type": "StatusResponse",
"attributes": {
"id": "5f148e12-fba3-432e-b0d5-fe3164axxxxx",
"status": "success",
"message": "rows_count=33490",
"rowCount": 33490,
"fileSizeBytes": 6669897,
"createdAt": "2025-02-14T14:59:20.000Z",
"expiresAt": "2025-02-21T15:00:00.000Z",
"md5CheckSum": "801993bc0fe04e2b3fcf767a1c867a04"
}
},
"warnings": [],
"errors": []
}
Reporting Asynchronous Workflow: Step 3 of 3
- Finally, download the report using the report output endpoint
- Report outputs are cached for at least 1 hour before expiration
- Exact expiration is indicated by the
expiresAt
field in the /status response
Download output of specific report
Once the report creation is completed ("status": "success"
in response above), the report output will be available to download in this endpoint.
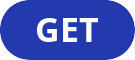
https://api.criteo.com/preview/retail-media/reports/{reportId}/output
Sample Request
curl -X GET "https://api.criteo.com/preview/retail-media/reports/2e733b8c-9983-4237-aab9-17a4xxxxxx/output" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
Sample Responses
[
{
"date": "2025-01-02",
"retailerName": "Retailer ABC",
"retailerId": 5317,
"placementId": 19389,
"placementName": "viewHome_API_mobile-Carousel",
"pageTypeName": "home",
"environment": "mobile",
"servedCategory": "shop",
"retailerCategoryId": 4215453,
"retailerCategoryName": "shop",
"pageViews": 330227,
"availablePlacements": 330235,
"unfilledPlacements": 260449,
"fillRate": 0.2198,
"placementImpressions": 72578,
"productImpressions": 115457,
"impressions": 115457,
"placementClicks": null,
"productClicks": 142,
"clicks": 142,
"placementImpressionsCTR": 0.001956515748573948,
"productImpressionsCTR": 0.001229895112466113,
"cpm": null,
"cpc": 0.19487323943662,
"placementImpressionsRevenue": 0,
"productClicksRevenue": 27.672,
"totalRevenue": 27.672,
"workingMedia": 34.59,
"netRevenue": 27.672,
"uniqueVisitors": 14536,
"frequency": 7.942831590533847001
},
// ...
{
"date": "2025-01-03",
"retailerName": "Retailer ABC",
"retailerId": 5317,
"placementId": 19389,
"placementName": "viewHome_API_mobile-Carousel",
"pageTypeName": "home",
"environment": "mobile",
"servedCategory": "shop",
"retailerCategoryId": 4215453,
"retailerCategoryName": "shop",
"pageViews": 250414,
"availablePlacements": 250417,
"unfilledPlacements": 203221,
"fillRate": 0.1936,
"placementImpressions": 48487,
"productImpressions": 73114,
"impressions": 73114,
"placementClicks": null,
"productClicks": 93,
"clicks": 93,
"placementImpressionsCTR": 0.001918039886980015,
"productImpressionsCTR": 0.00127198621331072,
"cpm": null,
"cpc": 0.198279569892473,
"placementImpressionsRevenue": 0,
"productClicksRevenue": 18.44,
"totalRevenue": 18.44,
"workingMedia": 23.05,
"netRevenue": 18.44,
"uniqueVisitors": 9983,
"frequency": 7.323850545928077732
}
]
Responses
Response | Description |
---|---|
🟢 200 | Call executed with success |
🔴 400 | Common Validation Errors: - Deserialization error - one or more input parameters is not supported; see details for more info - Please select an interval under 100 days - using a date range with more than 100 days apart between startDate and endDate - Time zone xyz is not valid - using a time zone value that is not listed in the list TZ database time zones |
🔴 403 | - You are not authorized to access some of the requested resources. - API user does not have the authorization to access some of the requested resources. Review the supply account IDs used in the request and, for an authorization request, follow the authorization request steps |
Updated 2 months ago