Open Auction Line Items
Getting Started
Learn more about how open auction line items work with our API Here!
Endpoints
Verb | Endpoint | Description |
---|---|---|
GET | /campaigns/{campaignId}/auction-line-items | Get all Open Auction Line items from a specific Campaign |
POST | /campaigns/{campaignId}/auction-line-items | Creates an Open Auction Line item |
GET | /auction-line-items/{lineItemId} | Get a specific Open Auction Line item |
PUT | /auction-line-items/{lineItemId} | Updates a specific Open Auction Line item |
Info
- Create operations using the
POST
method except every Required (R) field; omitting Optional (O) fields will set those fields to Default values- Update operations using the
PUT
method expect every Write (W) field; omitting these fields is equivalent to setting them tonull
, if possible
Line Item Attributes
Data Type: string
Description: Line Item ID
Accepted Value: int64
Default Values: None
Write? No
Nullable? No
campaignId Required
Data Type: string
Description: Campaign ID
Accepted Value: int64
Default Value: None
Write? No
Nullable? No
name Required
Data Type: string
Description: Line item name; must be unique within a campaign
Accepted Value: 255 char limit
Default Value: None
Write? Yes
Nullable? No
targetRetailerId Required
Data Type: string
Description: ID of the retailer the line item serves on
Accepted Value: int64
Default Value: None
Write? No
Nullable? No
startDate Required
Data Type: date
Description: Line item start date in the account timeZone
Accepted Value: YYYY-MM-DD
Default Value: None
Write? Yes
Nullable? No
endDate Optional
Data Type: date
Description: Line item end date in the account timeZone
; serves indefinitely if omitted or set to null
Accepted Value: YYYY-MM-DD
Default Value: null
Write? Yes
Nullable? Yes
budget Optional
Data Type: number
Description: Line item lifetime spend cap; uncapped if omitted or set to null
Accepted Value: at least 0
Default Value: null
Write? Yes
Nullable? Yes
budgetSpent
Data Type: number
Description: Amount the line item has already spent
Accepted Value: at least 0
Default Value: 0.0
Write? No
Nullable? No
budgetRemaining
Data Type: number
Description: Amount the line item has remaining until cap is hit; null
if budget is uncapped
Accepted Value: between 0 and budget
Default Value: null
Write? No
Nullable? Yes
monthlyPacing Optional
Data Type: number
Description: Amount the line item can spend per calendar month in the account timeZone
; resets each calendar month; uncapped if omitted or set to null
Accepted Value: at least 0
Default Value: null
Write? Yes
Nullable? Yes
dailyPacing Optional
Data Type: number
Description: Amount the line item can spend per day in the account timeZone; resets each day; overwritten by a calculation if isAutoDailyPacing
is configured; uncapped if omitted or set to null
Accepted Value: at least 0
Default Value: null
Write? Yes
Nullable? Yes
isAutoDailyPacing Required
Data Type: boolean
Description: To activate, either line item endDate
and budget, or monthlyPace
, must be specified; overwrites dailyPacing
with a calculation if not set prior
Accepted Value: true
, false
Default Value: false
Write? Yes
Nullable? No
bidStrategy Optional
Data Type: enum
Description: Bid algorithm optimizing for sales conversions, sales revenue, or clicks
Accepted Value: conversion
, revenue
, clicks
Default Value: conversion
Write? Yes
Nullable? No
targetBid Required
Data Type: number
Description: If optimizing for conversion
or revenue, a target average amount to bid because each bid is modulated up or down by our optimization algorithm; bids stay constant if optimizing for clicks
; must meet minBid
for line item to serve; minBid
depends on selected products and is retrieved through the catalog; input excludes platform fees
Accepted Value: at least the greatest value of minBid across all products on the line item
Default Value: 0.3
Write? Yes
Nullable? No
maxBid Optional
Data Type: number
Description: If optimizing for conversion
or revenue
, the maximum amount allowed to bid for each bid; respected regardless of targetBid
; must meet minBid
for line item to serve; minBid
depends on selected products and is retrieved through the catalog; bidding is uncapped if omitted or set to null
; does not apply if optimizing for clicks
; input excludes platform fees
Accepted Value: at least 0.01
Default Value: null
Write? Yes
Nullable? Yes
status
Data Type: enum
Description: Line item status; can only be updated by a user to active
or paused
; all other values are applied automatically depending on flight dates, financials, or missing attributes required for line item to serve. To understand the conditions that will cause a status to change, check out our status page.
Accepted Value: active
, paused
, scheduled
, ended
, budgetHit
, noFunds
, draft
, archived
Default Value: None
Write? No
Nullable? No
createdAt
Data Type: timestamp
Description: Timestamp in UTC of line item creation
Accepted Value: ISO-8601
Default Value: None
Write? No
Nullable? No
updatedAt
Data Type: timestamp
Description: Timestamp in UTC of last line item update
Accepted Value: ISO-8601
Default Value: None
Write? No
Nullable? No
Create an Open Auction Line Item
This endpoint creates a new open auction line item in the specified campaign.
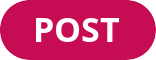
https://api.criteo.com/2023-04/retail-media/campaigns/{campaignId}/auction-line-items
Sample Request
curl -L -X POST 'https://api.criteo.com/2023-04/retail-media/campaigns/405429491681951744/auction-line-items' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
--data-raw '{
"data": {
"type": "405429491681951744",
"attributes": {
"name": "API Campaing",
"startDate": "2023-01-23",
"targetRetailerId": "299",
"endDate": "2023-01-31",
"status": "active",
"budget": "1.00",
"targetBid": "5",
"maxBid": "5",
"monthlyPacing": "50",
"dailyPacing": "5",
"isAutoDailyPacing": false,
"bidStrategy": "conversion"
}
}
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
"data": {
"type": "405429491681951744",
"attributes": {
"name": "API Campaing",
"startDate": "2023-01-23",
"targetRetailerId": "299",
"endDate": "2023-01-31",
"status": "active",
"budget": "1.00",
"targetBid": "5",
"maxBid": "5",
"monthlyPacing": "50",
"dailyPacing": "5",
"isAutoDailyPacing": False,
"bidStrategy": "conversion"
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
conn.request("POST", "/2023-04/retail-media/campaigns/405429491681951744/auction-line-items", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n\t\"data\": {\n\t\t\"type\": \"405429491681951744\",\n\t\t\"attributes\": {\n\t\t\t\"name\": \"API Campaing\",\n\t\t\t\"startDate\": \"2023-01-23\",\n\t\t\t\"targetRetailerId\": \"299\",\n\t\t\t\"endDate\": \"2023-01-31\",\n\t\t\t\"status\": \"active\",\n\t\t\t\"budget\": \"1.00\",\n\t\t\t\"targetBid\": \"5\",\n\t\t\t\"maxBid\": \"5\",\n\t\t\t\"monthlyPacing\": \"50\",\n\t\t\t\"dailyPacing\": \"5\",\n\t\t\t\"isAutoDailyPacing\": false,\n\t\t\t\"bidStrategy\": \"conversion\"\n\t\t}\n\t}\n}");
Request request = new Request.Builder()
.url("https://api.criteo.com/2023-04/retail-media/campaigns/405429491681951744/auction-line-items")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2023-04/retail-media/campaigns/405429491681951744/auction-line-items');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{\n "data": {\n "type": "405429491681951744",\n "attributes": {\n "name": "API Campaing",\n "startDate": "2023-01-23",\n "targetRetailerId": "299",\n "endDate": "2023-01-31",\n "status": "active",\n "budget": "1.00",\n "targetBid": "5",\n "maxBid": "5",\n "monthlyPacing": "50",\n "dailyPacing": "5",\n "isAutoDailyPacing": false,\n "bidStrategy": "conversion"\n }\n }\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"attributes": {
"name": "API Line Item",
"startDate": "2023-01-23",
"endDate": "2023-01-31",
"maxBid": 5.00000000,
"budget": 1.00000000,
"monthlyPacing": 50.00000000,
"dailyPacing": 5.00000000,
"bidStrategy": "conversion",
"targetRetailerId": "299",
"status": "draft",
"targetBid": 5.00000000,
"isAutoDailyPacing": false,
"campaignId": "405429491681951744",
"budgetSpent": 0.00000000,
"budgetRemaining": 1.00000000,
"createdAt": "2023-01-23T22:02:42+00:00",
"updatedAt": "2023-01-23T22:02:42+00:00",
"id": "405482503263227904"
},
"id": "405482503263227904",
"type": "RetailMediaAuctionLineItem"
},
"warnings": [],
"errors": []
}
Get All Open Auction Line Items
This endpoint lists all open auction line items in the specified campaign. Results are paginated
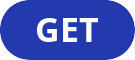
https://api.criteo.com/2023-04/retail-media/campaigns/{campaignId}/auction-line-items
Sample Request
curl -X GET "https://api.criteo.com/2023-04/retail-media/campaigns/8343086999167541140/auction-line-items" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
import requests
url = "https://api.criteo.com/2023-04/retail-media/campaigns/76216196459831296/auction-line-items"
payload={}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/2023-04/retail-media/campaigns/76216196459831296/auction-line-items")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2023-04/retail-media/campaigns/76216196459831296/auction-line-items');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"type": "RetailMediaLineItem",
"id": "9979917896105882144",
"attributes": {
"campaignId": "8343086999167541140",
"name": "Line Item 123",
"targetRetailerId": "3239117063738827231",
"startDate": "2020-04-06",
"endDate": null,
"budget": null,
"budgetSpent": 2383.87,
"budgetRemaining": null,
"monthlyPacing": null,
"dailyPacing": null,
"isAutoDailyPacing": false,
"bidStrategy": "conversion",
"targetBid": 1.50,
"maxBid": 2.50,
"status": "active",
"createdAt": "2020-04-06T17:29:11+00:00",
"updatedAt": "2020-04-06T17:29:11+00:00"
}
},
// ...
{
"type": "RetailMediaLineItem",
"id": "6854840188706902009",
"attributes": {
"campaignId": "8343086999167541140",
"name": "Line Item 789",
"targetRetailerId": "18159942378514859684",
"startDate": "2020-04-08",
"endDate": null,
"budget": 8000.00,
"budgetSpent": 1921.23,
"budgetRemaining": 6078.77,
"monthlyPacing": 1000.00,
"dailyPacing": 33.33,
"isAutoDailyPacing": true,
"bidStrategy": "conversion",
"targetBid": 0.75,
"maxBid": 1.25,
"status": "paused",
"createdAt": "2020-04-06T23:42:47+00:00",
"updatedAt": "2020-06-03T03:01:52+00:00"
}
}
],
"metadata": {
"totalItemsAcrossAllPages": 105,
"currentPageSize": 25,
"currentPageIndex": 0,
"totalPages": 5,
"nextPage": "https://api.criteo.com/2023-04/retail-media/campaigns/8343086999167541140/line-items?pageIndex=1&pageSize=25",
"previousPage": null
}
}
Get a Specific Open Auction Line Item
This endpoint retrieves the specified open auction line item
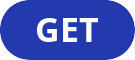
https://api.criteo.com/2023-04/retail-media/auction-line-items/{lineItemId}
Sample Request
curl -X GET "https://api.criteo.com/2023-04/retail-media/auction-line-items/2465695028166499188" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/2023-04/retail-media/auction-line-items/358669652976373760")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/2023-04/retail-media/auction-line-items/358669652976373760")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2023-04/retail-media/auction-line-items/358669652976373760');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaLineItem",
"id": "2465695028166499188",
"attributes": {
"campaignId": "8343086999167541140",
"name": "My New Line Item",
"targetRetailerId": "18159942378514859684",
"startDate": "2020-04-06",
"endDate": null,
"budget": null,
"budgetSpent": 0.00,
"budgetRemaining": null,
"monthlyPacing": null,
"dailyPacing": null,
"isAutoDailyPacing": false,
"bidStrategy": "conversion",
"targetBid": 0.30,
"maxBid": null,
"status": "draft",
"createdAt": "2020-04-06T06:11:23+00:00",
"updatedAt": "2020-04-06T06:11:23+00:00"
}
}
}
Update a Specific Open Auction Line Item
This endpoint updates the specified open auction line item. In this example, we enable auto daily pacing by setting a monthly pace simultaneously. Note that with auto daily pacing enabled, daily pacing is automatically calculated and overwrites its previous value, if any. Also, note the draft state of the line item because products to be promoted have not yet been added.
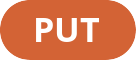
https://api.criteo.com/2023-04/retail-media/auction-line-items/{lineItemId}
Sample Request
curl -X PUT "https://api.criteo.com/2023-04/retail-media/auction-line-items/2465695028166499188" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>" \
-H "Content-Type: application/json" \
-d '{
"data": {
"type": "RetailMediaLineItem",
"id": "2465695028166499188",
"attributes": {
"name": "My New Line Item",
"startDate": "2020-04-06",
"endDate": null,
"budget": null,
"monthlyPacing": 30.00,
"dailyPacing": null,
"isAutoDailyPacing": true,
"bidStrategy": "conversion",
"targetBid": 0.30,
"maxBid": null,
"status": "active"
}
}
}'
import requests
import json
url = "https://api.criteo.com/2023-04/retail-media/auction-line-items/358669652976373760"
payload = json.dumps({
"data": {
"id": "358669652976373760",
"type": "RetailMediaCampaignUpdate",
"attributes": {
"name": "API Campaign Updated new name",
"startDate": "2022-09-16",
"targetRetailerId": "299",
"endDate": "2022-09-18",
"status": "draft",
"budget": "35",
"targetBid": "0.3",
"maxBid": "0.10",
"monthlyPacing": "50",
"isAutoDailyPacing": True,
"bidStrategy": "conversion"
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("PUT", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"data\": {\n \"id\": \"358669652976373760\",\n \"type\": \"RetailMediaCampaignUpdate\",\n \"attributes\": {\n \"name\": \"API Campaign Updated\",\n \"startDate\": \"2022-09-16\",\n \"targetRetailerId\": \"299\",\n \"endDate\": \"2022-09-18\",\n \"status\": \"active\",\n \"budget\": \"15\",\n \"targetBid\": \"0.3\",\n \"maxBid\": \"0.10\",\n \"monthlyPacing\": \"50\",\n \"isAutoDailyPacing\": true,\n \"bidStrategy\": \"conversion\"\n }\n }\n}");
Request request = new Request.Builder()
.url("https://api.criteo.com/2023-04/retail-media/auction-line-items/358669652976373760")
.method("PUT", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/2023-04/retail-media/auction-line-items/358669652976373760');
$request->setMethod(HTTP_Request2::METHOD_PUT);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{\n "data":
{\n "id": "358669652976373760",
\n "type": "RetailMediaCampaignUpdate",
\n "attributes": {
\n "name": "API Campaign Updated",
\n "startDate": "2022-09-16",
\n "targetRetailerId": "299",
\n "endDate": "2022-09-18",
\n "status": "active",
\n "budget": "15",
\n "targetBid": "0.3",
\n "maxBid": "0.10",
\n "monthlyPacing": "50",
\n "isAutoDailyPacing": true,
\n "bidStrategy": "conversion"
\n }
\n }
\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaLineItem",
"id": "2465695028166499188",
"attributes": {
"campaignId": "8343086999167541140",
"name": "My New Line Item",
"targetRetailerId": "18159942378514859684",
"startDate": "2020-04-06",
"endDate": null,
"budget": null,
"budgetSpent": 0.00,
"budgetRemaining": null,
"monthlyPacing": 3000.00,
"dailyPacing": 120.00,
"isAutoDailyPacing": true,
"bidStrategy": "conversion",
"targetBid": 0.30,
"maxBid": null,
"status": "draft",
"createdAt": "2020-04-06T06:11:23+00:00",
"updatedAt": "2020-04-06T06:17:48+00:00"
}
}
}
Responses
Responses | Description |
---|---|
🔵 200 | Call completed with success |
🔵 201 | Line item created with success |
🔴 400 | Bad request leading to a validation error Common validation errors - Invalid is auto daily pacing - Cannot turn on IsAutoDailyPacing and add a DailyPacing value. Only one of the two options can be used |
Updated almost 2 years ago