Negative Keyword Targeting
Future Deprecation
The following endpoints will soon be replaced by the new Keywords API. You may continue using the following endpoints without disrupting your services. We recommend reviewing and testing the new Keyword endpoints to prepare for a future migration to these new endpoints.
A FEW THINGS TO KNOW
For an overview, read more about how Negative Keyword Targeting works in our Help Center
Endpoints
Verb | Endpoint | Description |
---|---|---|
GET | /auction-line-items/{line-item-id}/targeting/keywords | Get All Negated Keywords on a specific Line Item |
POST | /auction-line-items/{line-item-id}/targeting/keywords/append | Add Negated Keywords to a Specific Line Item |
POST | /auction-line-items/{line-item-id}/targeting/keywords/delete | Remove Negated Keywords from a Specific Line Item |
Negated Keyword Attributes
Data Type: string
Description: Keyword and match-type
Values: Dictionary of keyword to matchType enum; possible matchType values are negativeExact
and negativeBroad
Get all Negated Keywords on a Line item
This endpoint list all negated keywords to an Open Auction Line item
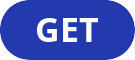
https://api.criteo.com/preview/retail-media/auction-line-items/{line-item-id}/targeting/keywords
Sample Request
curl -X GET "https://api.criteo.com/preview/retail-media/auction-line-items/2465695028166499188/targeting/keywords" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
import requests
url = "https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords"
payload={}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
Sample Response
{
"data": {
"type": "RetailMediaKeywordTarget",
"id": "2465695028166499188",
"attributes": {
"keywords": {
"chips": "negativeExact",
"soda": "negativeBroad"
}
}
},
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Add Negated Keywords on a Line item
This endpoint adds negated keywords to an Open Auction Line item
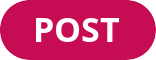
https://api.criteo.com/preview/retail-media/auction-line-items/{line-item-id}/targeting/keywords/append
Sample Request
curl -X POST "https://api.criteo.com/preview/retail-media/auction-line-items/2465695028166499188/targeting/keywords/append" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>" \
-H "Content-Type: application/json" \
-d '{
"data": [
{
"type": "RetailMediaKeywordTarget",
"attributes": {
"keywords": {
"chips": "negativeExact",
"soda": "negativeBroad"
}
},
]
}
import requests
import json
url = "https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords/append"
payload = json.dumps({
"data": {
"type": "<string>",
"attributes": {
"keywords": {
"pasta": "NegativeExact",
"juice": "NegativeBroad"
}
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"data\": {\n \"type\": \"<string>\",\n \"attributes\": {\n \"keywords\": {\n \"pasta\": \"NegativeExact\",\n \"juice\": \"NegativeBroad\"\n }\n }\n }\n}");
Request request = new Request.Builder()
.url("https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords/append")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords/append');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{\n "data": {\n "type": "<string>",\n "attributes": {\n "keywords": {\n "pasta": "NegativeExact",\n "juice": "NegativeBroad"\n }\n }\n }\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaKeywordTarget",
"id": "2465695028166499188",
"attributes": {
"keywords": {
"chips": "negativeExact",
"soda": "negativeBroad"
}
}
},
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Remove Negated Keywords on a Line Item
This endpoint removes previously negated keywords (as a result, these keywords might be targeted)
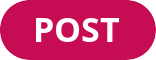
https://api.criteo.com/preview/retail-media/auction-line-items/{line-item-id}/targeting/keywords/delete
Sample Request
curl -X POST "https://api.criteo.com/preview/retail-media/auction-line-items/2465695028166499188/targeting/keywords/delete" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>" \
-H "Content-Type: application/json" \
-d '{
"data": [
{
"type": "RetailMediaPromotedProduct",
"attributes": {
"keywords": {
"chips": "negativeExact"
}
}
}
]
}
import requests
import json
url = "https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords/delete"
payload = json.dumps({
"data": {
"type": "<string>",
"attributes": {
"keywords": {
"apple": "negativeBroad",
"juice": "NegativeBroad"
}
}
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"data\": {\n \"type\": \"<string>\",\n \"attributes\": {\n \"keywords\": {\n \"apple\": \"negativeBroad\",\n \"juice\": \"NegativeBroad\"\n }\n }\n }\n}");
Request request = new Request.Builder()
.url("https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords/delete")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/preview/retail-media/auction-line-items/325713346766241792/targeting/keywords/delete');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
$request->setBody('{\n "data": {\n "type": "<string>",\n "attributes": {\n "keywords": {\n "apple": "negativeBroad",\n "juice": "NegativeBroad"\n }\n }\n }\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"type": "RetailMediaPromotedProduct",
"attributes": {
"keywords": {
"chips": "negativeBroad"
}
}
}
],
"metadata": {
"totalItemsAcrossAllPages": 4,
"currentPageSize": 4,
"currentPageIndex": 0,
"totalPages": 1,
"nextPage": null,
"previousPage": null
}
}
Responses
Response | Description |
---|---|
🔵 200 | Call completed with success |
🔴 400 | Bad request, validation error |
Updated about 1 month ago