OAuth App implementation
Introduction
The Criteo API allows you to build custom apps that help the world's advertisers grow their businesses.
In order for your app to function, your users will have to delegate permissions for one or more of the Criteo advertisers that they oversee.
To do this, you will need to direct the user to a unique consent delegation page using a standard OAuth integration (Authorization Code Grant Type). Below we describe how to implement this flow.
These guides assume you have created a developer account, are part of an organization. Instructions for getting started can be found here.
Creating an Authorization code app
Once you have logged in to the Criteo Partners Portal, create a new App by clicking on "+" in the My apps section.
This will open a modal where you can select type of application. To create an OAuth app use the "Authorization code" app.
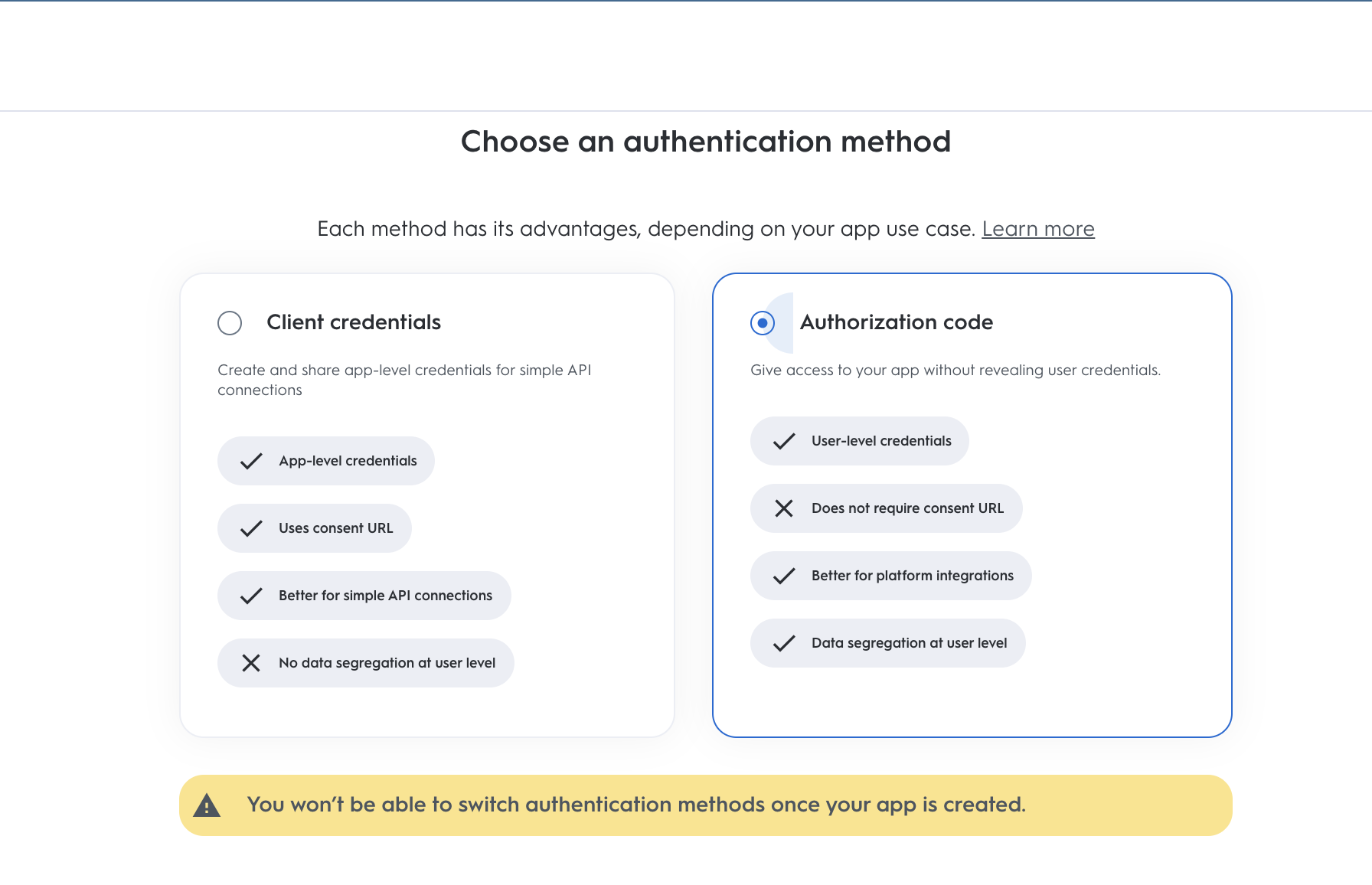
Define the name, description and image of your app. This will create your App page.
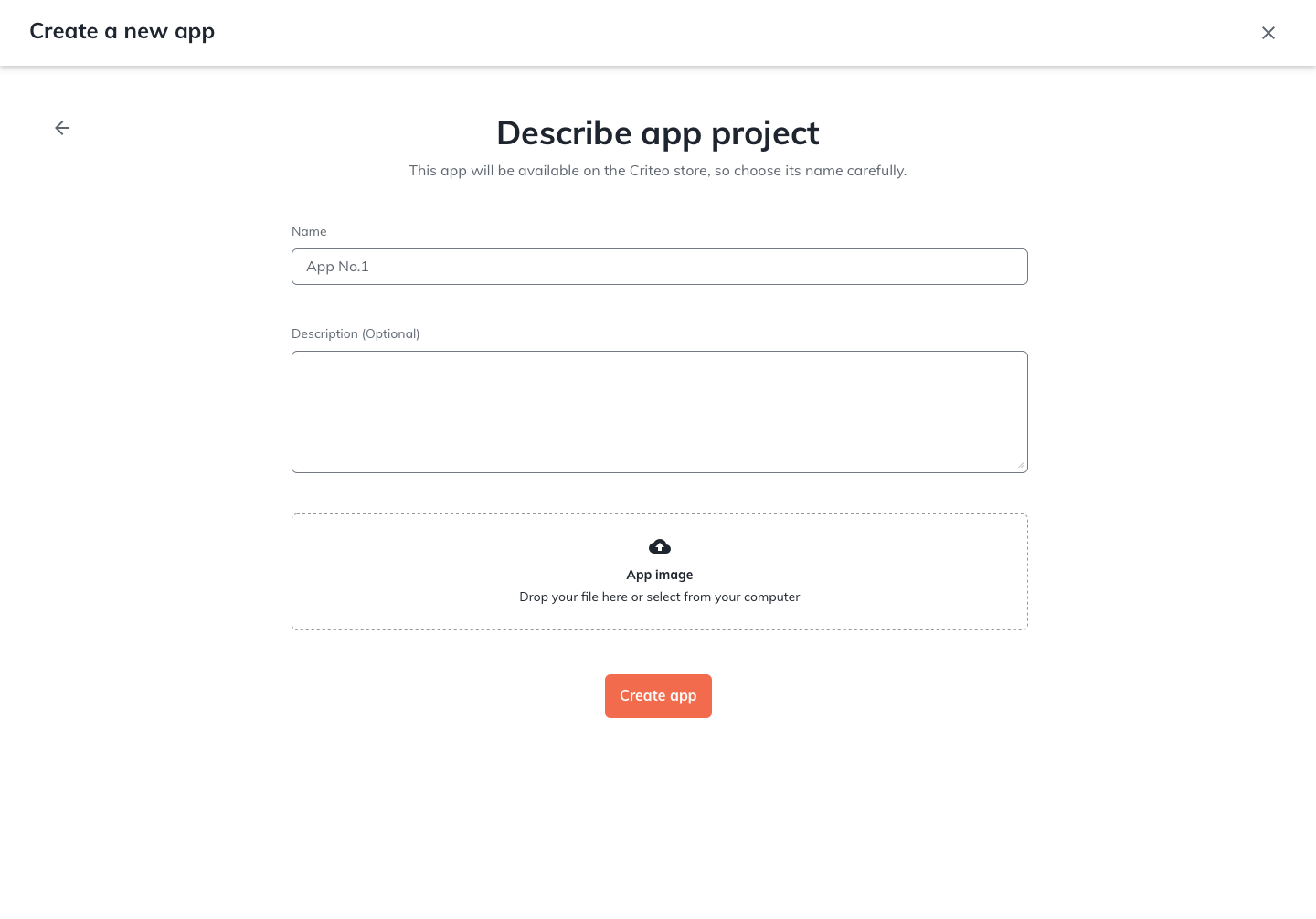
In your App page you will be able to define the scope of your application and the OAuth parameters. Please see this page to see more details on how to define your app scope.
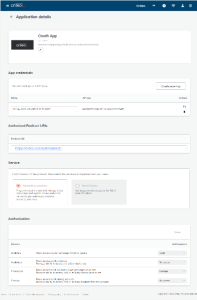
Set up your OAuth parameters
Once you have defined the scope of your application, you will be able to setup what you need for the OAuth workflow
Parameters | Description |
---|---|
client_id | Your public key accessible in app credentials section. You can add up to 5 pairs of credentials. Can be added and managed also once the App has been activated. |
client_secret | Your secret key, accessible only once when creating a pair of client_id and client_secret in credentials section. You can add up to 5 pairs of credentials. Can be added and managed also once the App has been activated. |
redirect_uri | The URL to redirect the user to after consent delegation. Https protocol required. You can add up to 30 redirect URIs. Can be added and managed also once the App has been activated. |
You can now publish the app, and start an authorization code workflow.
Consent URL creation
Once you have defined the parameters of your App you can start implementing your authorization code flow.
To request access to a user to access their data, you will create a Consent link (to be sent to the user or implemented through a button in your platform that will redirect the user to the link) with the following structure and parameters:
https://consent.criteo.com/request
?response_type=code
&client_id=6e75a
&redirect_uri=https%3A%2F%2Fyourwebsite.com%2Fauth%2Fredirect
&state=4lr4e
Parameters | Description |
---|---|
response_type=code | Indicates that an authorization code is expected as outcome. |
client_id | Your public key accessible in app credentials section. |
redirect_uri | The URL to redirect the user to after consent delegation. Https protocol required. Defined in the redirect URI section in Developer Dashboard. |
state | A string that you can provide and that will be returned as-is in the final redirection (usually used to prevent Cross-Site Request Forgery attacks). |
The Consent link will direct your user to Criteo Consent page:
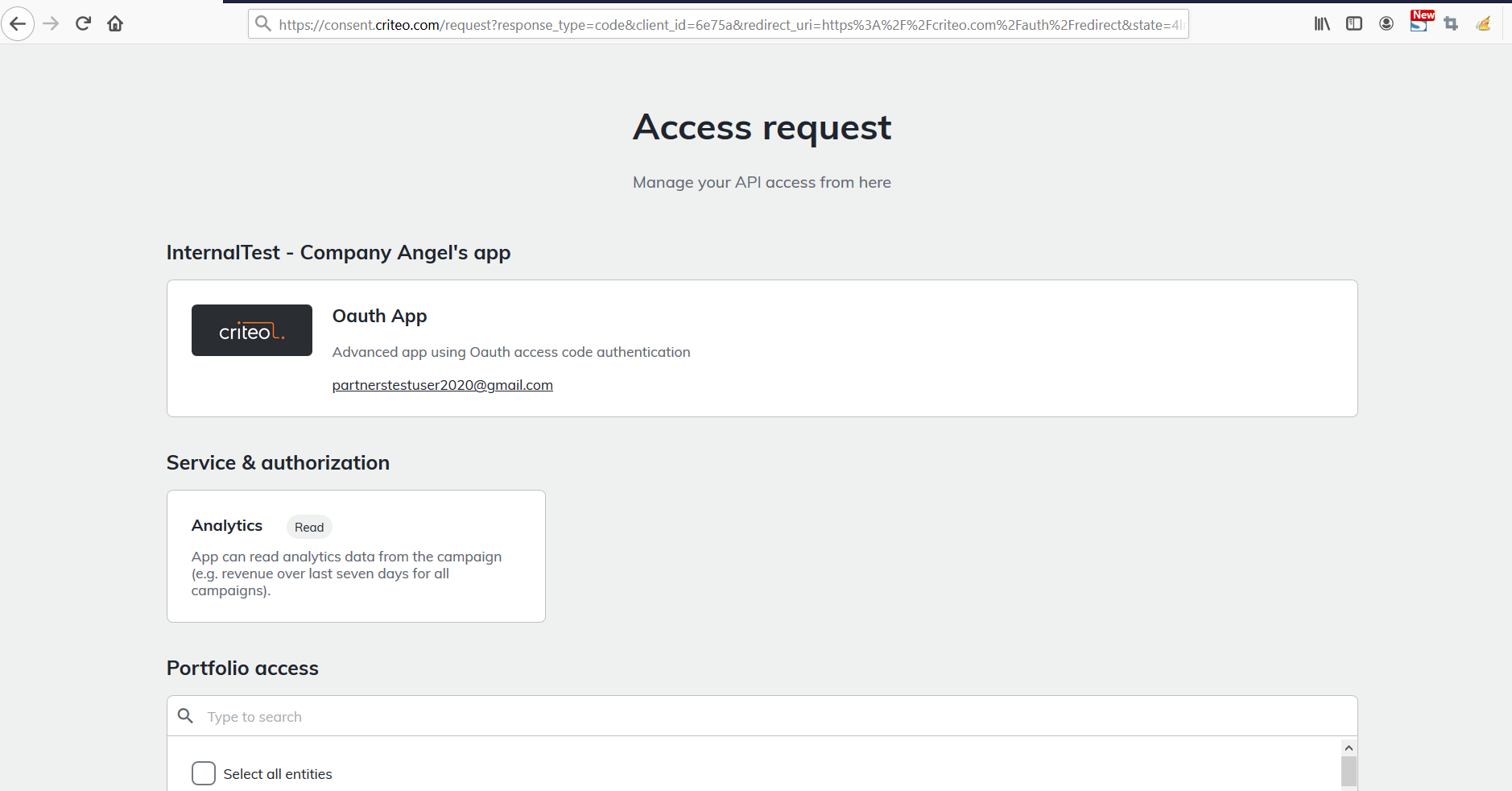
They can choose what advertisers from their portfolio they want to give access to.
They approve the request by clicking on "Approve".
A consent request is displayed to a user in all cases except in the following:
- The client_id does not match any published API app.
- The redirect_uri is not authorized (e.g not accessible from public network)
- An unexpected error occurred in our backend.
In any of the cases above an error message will be displayed.
Redirection and access code
Once the user has completed the Consent Delegation flow, we redirect your user to the following URL:
https://yourwebsite.com/auth/redirect
?code=eyJ.abc.def
&state=4lr4e
This URL is forged with the authorized redirect_uri and the following query parameters:
Parameter | Description |
---|---|
code | An authorization code valid for 30 seconds, usable only once. |
state | The state parameter that you originally provided (returned as-is). |
If the consent request is denied, we redirect to the same redirect-uri but with an 'error' query parameter instead of a 'code'.
Exchanging access code for access token
Now that you have an authorization code, you can trade it for an access token with the following request:
POST https://api.criteo.com/oauth2/token
grant_type=authorization_code
&code=eyJ.abc.def
&redirect_uri=https://yourwebsite.com/auth/redirect
&client_id=6e75a
&client_secret=j7MfAp
Parameter | Description |
---|---|
grant_type=authorization_code | Indicates that you are providing an authorization code. |
code | Authorization code returned during redirection. |
redirect_uri | Must match the redirect_uri used for the authorization request. |
client_id | Your public key accessible in app credentials section. |
client_secret | Your secret key, accessible only once when creating a pair of client_id and client_secret in credentials section. |
The response from Criteo API will be the following:
{
"access_token": "eyJ.fiZfef.dls",
"refresh_token": "eyJ.ela4a9e.afd",
"token_type": "Bearer",
"expires_in": 900
}
Parameter | Description |
---|---|
access_token | A short-lived (valid for 900 seconds) access token* |
refresh_token | A long-lived refresh token (that expires after 6 months) that can be used to renew the access token (see next section). |
token_type=Bearer | Type of token. |
expires_in | Lifetime of the token in seconds. |
Note about token lifetime
The refresh token will be revoked if the user that provided consent to an account changes the role or leaves the organization. Such an account needs to be re-authorized by launching the new consent flow and having the new administrator accept the consent.
Using refresh token
When an access token is close to expiration, you can get a new one using a refresh token via the following request:
POST https://api.criteo.com/oauth2/token
grant_type=refresh_token
&refresh_token=eyJ.ela4a9e.afd
&client_id=6e75a
&client_secret=j7MfAp
Parameter | Description |
---|---|
grant_type=refresh_token | Indicates that you are providing a refresh token. |
refresh_token | Refresh token shared when requesting an access token. |
client_id | Your public key accessible in app credentials section. |
client_secret | Your secret key, accessible only once when creating a pair of client_id and client_secret in credentials section. |
The response will be the same as when issuing an access token
Demo
Below you can find a code for a demo application in NodeJS that uses Express JS framework.
var express = require('express');
var passport = require('passport');
var OAuth2Strategy = require('passport-oauth2').Strategy;
var app = express();
var port = 3000;
// Passport setup
passport.use(new OAuth2Strategy({
clientID: 'CLIENT_ID', // Enter your client_id here
clientSecret: 'CLIENT_SECRET', // Enter your client_secret here
authorizationURL: 'https://consent.criteo.com/request',
callbackURL: `http://localhost:${port}/criteo-auth/callback`,
tokenURL: 'https://api.criteo.com/oauth2/token',
state: 'togorot'
},
function(accessToken, refreshToken, profile, cb) {
cb(null, { accessToken, refreshToken });
}
));
app.use(passport.initialize());
// Route declarations
app.get('/', function(req, res) {
res.send('<a href="/criteo-auth">Link my Criteo account!</a>');
});
app.get('/criteo-auth', passport.authenticate('oauth2'));
app.get('/criteo-auth/callback',
passport.authenticate('oauth2', { session: false }),
function(req, res) {
res.send(`<div>Authentication successful!</div><div>Access token:</div><textarea>${req.user.accessToken}</textarea><div>Refresh token:</div><textarea>${req.user.refreshToken}</textarea>`);
}
);
console.log(`OAuth test app started on http://localhost:${port}`);
app.listen(port);
{
"name": "oauth-node",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"ejs": "^3.1.6",
"express": "^4.17.1",
"passport": "^0.4.1",
"passport-oauth2": "^1.6.0"
}
}
How to run the demo?
- Run
npm install
- Connect to the developer portal and create an app according to the instructions above
- Create an "Authorization code" app.
- Generate app credentials and enter the client_id and client_secret in index.js.
- Register "http://localhost:3000/criteo-auth/callback" as the redirect URI.
- Run
npm run start
- Open http://localhost:3000
FAQ
What happens if my client_id and client_secret are compromised?
You will need to delete the set of credentials in the App page and create a new one. You will need to request access again to your users.
Updated about 1 month ago