Audience Segment Endpoints
Endpoints
Verb | Endpoint | Description |
---|---|---|
POST | /retail-media/accounts/{account-id}/audience-segments/create | Create a new Audience Segment |
PATCH | /retail-media/accounts/{account-id}/audience-segments | Update an Audience Segment |
POST | /retail-media/accounts/{account-id}/audience-segments/delete | Delete an Audience Segment |
POST | /retail-media/accounts/{account-id}/audience-segments/search | Returns a list of segments that match the provided filters. If present, the filters are AND'ed together when applied. You can search segments by segment IDs, retailer IDs and/or segment types |
GET | /retail-media/accounts/{account-id}/audience-segments/{audience-segment-id}/contact-list | Retrieve contact list statistics |
POST | /retail-media/audience-segments/{audience-segment-id}/contact-list/add-remove | Add/remove identifiers in contact list Audience Segment |
POST | /retail-media/audience-segments/{audience-segment-id}/contact-list/clear | Clear all identifiers in contact list Audience Segment |
Audience Segment Attributes
Attribute | Data Type | Description |
---|---|---|
id | string | Audience Segment ID, generated internally by Criteo Accepted values: string of int64 Writeable? N / Nullable? N |
name | string | Audience Segment name Accepted values: string Writeable? Y / Nullable? N |
description | string | Description of the Audience Segment Accepted values: string Writeable? Y / Nullable? N |
accountId | string | Account ID associated with the Audience Segment, generated internally by Criteo Accepted values: string of int64 Writeable? N / Nullable? N |
retailerId * | string | Retailer ID, associated with the Audience Segment, generated internally by Criteo Accepted values: string of int64 Writeable? N / Nullable? N |
channels | list | Channels associated to the audience Accepted values: Onsite , Offsite , Unknown Writeable? N / Nullable? N |
contactList | object | Setting to target users with contact list. Note, either one of contactList or events is required, however both cannot be leveraged at the same timeSee below for more details |
events | object | Settings to target users based on their events. Note, either one of contactList or events is required, however both cannot be leveraged at the same timeSee below for more details |
createdAt | timestamp | Timestamp of Audience Segment creation, in UTC Accepted values: yyyy-mm-ddThh:mm:ss.msZ (in ISO-8601 )Writeable? N / Nullable? N |
createdById | string | User ID who created the Audience Segment (null if created by a service)Accepted values: string Writeable? N / Nullable? Y |
updatedAt | timestamp | Timestamp of last Audience Segment update, in UTC Accepted values: yyyy-mm-ddThh:mm:ss.msZ (in ISO-8601)Writeable? N / Nullable? N |
* Required at create operation
Contact List Attributes
Attribute | Data Type | Description |
---|---|---|
IsReadOnly | boolean | Indicates if the contact list can be edited Accepted values: true , false Writeable? N / Nullable? N |
identifierType | enum | User identifier type from Contact list Accepted values: Email , UserIdentifier , IdentityLink , CustomerId , Unknown Writeable? N / Nullable? N |
Events Attributes
Attribute | Data Type | Description |
---|---|---|
shopperActivity | enum | Define users who performed specific action Accepted values: View , Buy , AddToCart , Unknown Writeable? N / Nullable? N |
lookbackDays | enum | Define users who interact with the website in the respective recent timeframe Accepted values: Last7Days , Last14Days , Last30Days , Last45Days , Last60Days , Last90Days , Last120Days , Last150Days , Last180Days Writeable? N / Nullable? N |
categoryIds | list | Define users interested in specific categories from retailer's Catalog. Note, either one of categoryIds or brandIds must be included. Both can be included as well, but the request will fail if neither are includedAccepted values: array of strings/int64 Writeable? N / Nullable? Y |
brandIds | list | Define users interested in specific brands from retailer's Catalog. Note, either one of categoryIds or brandIds must be included. Both can be included as well, but the request will fail if neither are includedAccepted values: array of strings/int64 Writeable? N / Nullable? Y |
minPrice | decimal | Define users who interact with products whose prices are greater than minPrice Accepted values: minPrice ≥ 0.0Writeable? Y / Nullable? Y |
maxPrice | decimal | Define users who interact with products whose prices are smaller than maxPrice Accepted values: maxPrice ≤ 0.0Writeable? Y / Nullable? Y |
Create Audience Segment
This endpoints allows to create Audience Segments, either from Contact List or Users Events type.
Note: the corresponding App should have the "Audiences Manage" permission enabled.
As of now, it is only possible to create Contact List segments through our API. For Users Events Segments, users can rely on the C-Max UI - for more details, check (Onsite Display) Build an Audience
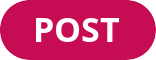
https://api.criteo.com/{version}/retail-media/accounts/{account-id}/audience-segments/create
Sample Request
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/create' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": [
{
"type": "AudienceSegment",
"attributes": {
"name": "Segment Name",
"description": "Segment Description",
"retailerId": "1234",
"contactList": {
"identifierType": "Email"
}
}
}
]
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
{
"data": [
{
"type": "AudienceSegment",
"attributes": {
"name": "Segment Name",
"description": "Segment Description",
"retailerId": "1234",
"contactList": {
"identifierType": "Email"
}
}
}
]
}
})
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("POST", "/{version}/retail-media/accounts/625702934721171442/audience-segments/create", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":[{"identifiertype":"AudienceSegment","attributes":{"name":"Segment Name","description":"Segment Description","retailerId":"1234","contactList":{"identifierType":"Email"}}}]}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/create")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/create');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":[{"type":"AudienceSegment","attributes":{"name":"Segment Name","description":"Segment Description","retailerId":"1234","contactList":{"identifierType":"Email"}}}]}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"id": "225702933721171456",
"type": "AudienceSegment",
"attributes": {
"name": "Segment Name",
"type": "ContactList",
"createdAt": "2024-01-23T09:33:40.822Z",
"updatedAt": "2024-01-23T09:33:40.822Z",
"accountId": "625702934721171442",
"retailerId": "1234",
"contactList": {
"isReadOnly": "true",
"identifierType": "Email"
}
}
}
],
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Update Audience Segment
This endpoints allows to update Audience Segments. Currently, it's only possible update Contact List segments.
Note: the corresponding App should have the "Audiences Manage" permission enabled.
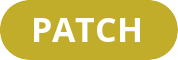
https://api.criteo.com/{version}/retail-media/accounts/{account-id}/audience-segments
Sample Request
curl -L -X PATCH 'https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": [
{
"attributes": {
"name": "New Name",
"description": {
"value": "New Description"
},
"contactList": {
}
},
"id": "225702933721171456",
"identifierType": "AudienceSegment"
}
]
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps(
{
"data": [
{
"attributes": {
"name": "New Name",
"description": {
"value": "New Description"
},
"contactList": {
}
},
"id": "1001",
"identifierType": "AudienceSegment"
}
]
)
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("PATCH", "/{version}/retail-media/accounts/625702934721171442/audience-segments", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":[{"attributes":{"name":"New Name","description":{"value":"New Description"},"contactList":{}},"id":"1001","identifierType":"AudienceSegment"}]}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments")
.method("PATCH", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments');
$request->setMethod(HTTP_Request2::METHOD_PATCH);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":[{"attributes":{"name":"New Name","description":{"value":"New Description"},"contactList":{}},"id":"1001","identifierType":"AudienceSegment"}]}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"id": "225702933721171456",
"type": "AudienceSegment",
"attributes": {
"name": "New Name",
"description": "New Description",
"type": "ContactList",
"createdAt": "2024-01-23T09:33:40.822Z",
"updatedAt": "2024-01-23T09:33:40.822Z",
"accountId": "625702934721171442",
"retailerId": "1234",
"contactList": {
"isReadOnly": "true",
"identifierType": "Email"
}
}
}
],
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Delete Audience Segment
This endpoints allows to delete Audience Segments.
Note: the corresponding App should have the "Audiences Manage" permission enabled.
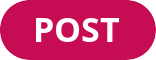
https://api.criteo.com/{version}/retail-media/accounts/{account-id}/audience-segments/delete
Sample Request
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/delete' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": [
{
"id": "225702933721171456",
"identifierType": "Audience Segment"
}
]
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
{
"data": [
{
"id": "225702933721171456",
"identifierType": "Audience Segment"
}
]
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("POST", "/{version}/retail-media/accounts/625702934721171442/audience-segments/delete", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":[{"id":"225702933721171456","identifierType":"Audience Segment"}]}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/delete")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/delete');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":[{"id":"225702933721171456","identifierType":"Audience Segment"}]}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"id": "225702933721171456",
"identifierType": "Audience Segment"
}
],
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Search for Audience Segments
This endpoints allows search for existing Audience Segments that satisfy one or few attributes at the same time.
Results are paginated using offset
and limit
query parameters; if omitted, defaults to 0
and 500
, respective - see API Response
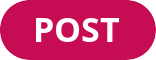
https://api.criteo.com/{version}/retail-media/accounts/{account-id}/audience-segments/search?offset={offset}&limit={limit)
Sample Request: searching by Retailer ID only
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": {
"type": "AudienceSegment",
"attributes": {
"audienceSegmentIds": null,
"retailerIds": [
"12"
],
"audienceSegmentTypes": null
}
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
{
"data": {
"type": "AudienceSegment",
"attributes": {
"audienceSegmentIds": null
"retailerIds": [
"12"
],
"audienceSegmentTypes": null
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("POST", "/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":{"type":"AudienceSegment","attributes":{"audienceSegmentIds":null,"retailerIds":["12"],"audienceSegmentTypes":null}}}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":{"type":"AudienceSegment","attributes":{"audienceSegmentIds":null,"retailerIds":["12"],"audienceSegmentTypes":null}}}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"meta": {
"totalItems": 400,
"limit": 50,
"offset": 0
},
"data": [
{
"id": "203134567785554216",
"type": "AudienceSegment",
"attributes": {
"name": "Segment Name",
"type": "Events",
"createdAt": "2024-01-15T12:23:18.180Z",
"updatedAt": "2024-01-15T12:23:18.180Z",
"accountId": "625702934721171442",
"retailerId": "12",
"events": {
"shopperActivity": "View",
"lookbackDays": "Last90Days",
"categoryIds": [
"3590922"
],
"brandIds": []
}
}
},
// ...
{
"id": "225702933721171456",
"type": "AudienceSegment",
"attributes": {
"name": "Segment Name",
"type": "ContactList",
"createdAt": "2024-01-23T09:33:40.822Z",
"updatedAt": "2024-01-23T09:33:40.822Z",
"accountId": "625702934721171442",
"retailerId": "12",
"contactList": {
"isReadOnly": "true",
"identifierType": "Email"
}
}
}
],
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Sample Request: searching by multiple filters
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": {
"type": "AudienceSegment",
"attributes": {
"audienceSegmentIds": ["225702933721171456"],
"retailerIds": [
"12"
],
"audienceSegmentTypes": ["ContactList"]
}
}
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
{
"data": {
"type": "AudienceSegment",
"attributes": {
"audienceSegmentIds": ["225702933721171456"],
"retailerIds": [
"12"
],
"audienceSegmentTypes": ["ContactList"]
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("POST", "/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":{"type":"AudienceSegment","attributes":{"audienceSegmentIds":["225702933721171456"],"retailerIds":["12"],"audienceSegmentTypes":["ContactList"]}}}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/search?limit=50&offset=0');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":{"type":"AudienceSegment","attributes":{"audienceSegmentIds":["225702933721171456"],"retailerIds":["12"],"audienceSegmentTypes":["ContactList"]}}}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"meta": {
"totalItems": 400,
"limit": 50,
"offset": 0
},
"data": [
{
"id": "225702933721171456",
"type": "AudienceSegment",
"attributes": {
"name": "Segment Name",
"type": "ContactList",
"createdAt": "2024-01-23T09:33:40.822Z",
"updatedAt": "2024-01-23T09:33:40.822Z",
"accountId": "625702934721171442",
"retailerId": "12",
"contactList": {
"isReadOnly": "true",
"identifierType": "Email"
}
}
}
],
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Get Contact List Segment Statistics
This endpoints allows to retrieve statistics from Contact List segments.
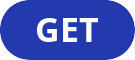
https://api.criteo.com/{version}/retail-media/accounts/{account-id}/audience-segments/{audience-segment-id}/contact-list
Sample Request
curl -L -X GET 'https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/225702933721171456/contact-list' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>'
import http.client
conn = http.client.HTTPSConnection("api.criteo.com")
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
conn.request("GET", "/{version}/retail-media/accounts/625702934721171442/audience-segments/225702933721171456/contact-list", headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/225702933721171456/contact-list")
.method("GET")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/625702934721171442/audience-segments/225702933721171456/contact-list');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"attributes": {
"numberOfIdentifiers": 10000,
"numberOfMatches": 5000,
"matchRate": 0.5
},
"id": "225702933721171456",
"identifierType": "AudienceSegment"
},
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Add/Remove identifiers in Contact List Audience Segment
This endpoint allows to add/remove users in a specific Contact List segment.
Note: the corresponding App should have the "Audiences Manage" permission enabled.
Attribute | Data Type | Description |
---|---|---|
operation | enum | Operation required for the sub-set of users provided in the request Accepted values: add , remove Writeable? N / Nullable? N |
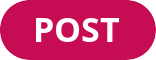
https://api.criteo.com/{version}/retail-media/audience-segments/{audience-segment-id}/contact-list/add-remove
Sample Request - adding users to existing audience segment
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": {
"type": "AddRemoveContactlist",
"attributes": {
"operation": "add",
"identifierType": "email",
"identifiers": [
"[email protected]",
"[email protected]",
"[email protected]"
]
}
}
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
{
"data": {
"type": "AddRemoveContactlist",
"attributes": {
"operation": "add",
"identifierType": "email",
"identifiers": [
"[email protected]",
"[email protected]",
"[email protected]",
]
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("POST", "/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":{"type":"AddRemoveContactlist","attributes":{"operation":"add","identifierType":"email","identifiers":["[email protected]","[email protected]","[email protected]"]}}}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":{"type":"AddRemoveContactlist","attributes":{"operation":"add","identifierType":"email","identifiers":["[email protected]","[email protected]","[email protected]"]}}}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "AddRemoveContactlistResult",
"attributes": {
"contactListId": 523103620165619700,
"operation": "add",
"requestDate": "2024-04-22T14:29:07.994Z",
"identifierType": "email",
"nbValidIdentifiers": 3,
"nbInvalidIdentifiers": 0,
"sampleInvalidIdentifiers": []
}
},
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Sample Request - removing users from existing audience segment
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>' \
-d '{
"data": {
"type": "ContactlistAmendment",
"attributes": {
"operation": "remove",
"identifierType": "email",
"identifiers": [
"[email protected]"
]
}
}
}'
import http.client
import json
conn = http.client.HTTPSConnection("api.criteo.com")
payload = json.dumps({
{
"data": {
"type": "ContactlistAmendment",
"attributes": {
"operation": "remove",
"identifierType": "email",
"identifiers": [
"[email protected]"
]
}
}
}
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Bearer <TOKEN>'
}
conn.request("POST", "/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data":{"type":"ContactlistAmendment","attributes":{"operation":"remove","identifierType":"email","identifiers":["[email protected]"]}}}');
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/add-remove');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json',
'Accept' => 'application/json',
'Authorization' => 'Bearer <TOKEN>'
));
$request->setBody('{"data":{"type":"ContactlistAmendment","attributes":{"operation":"remove","identifierType":"email","identifiers":["[email protected]"]}}}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "ContactlistAmendment",
"attributes": {
"operation": "remove",
"requestDate": "2018-12-10T10:00:50.000Z",
"identifierType": "email",
"nbValidIdentifiers": 7342,
"nbInvalidIdentifiers": 13,
"sampleInvalidIdentifiers": [
"InvalidIdentifier"
]
}
},
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Identifier List Size Limit
Note that there is a limit of 50,000 identifiers per single request. If you are adding more than 50,000 users, please split them into chunks of 50,000 and make multiple requests.
Clear all identifiers in Contact List Audience Segment
This endpoints resets a Contact List segment, erasing all existing users identifiers.
Note: the corresponding App should have the "Audiences Manage" permission enabled.
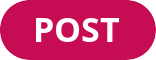
https://api.criteo.com/{version}/retail-media/audience-segments/{audience-segment-id}/contact-list/clear
Sample Request
curl -L -X POST 'https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/clear' \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer <MY_ACCESS_TOKEN>'
import http.client
conn = http.client.HTTPSConnection("api.criteo.com")
payload = ''
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
conn.request("POST", "/{version}/retail-media/audience-segments/225702933721171456/contact-list/clear", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/clear")
.method("POST", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/audience-segments/225702933721171456/contact-list/clear');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "ContactListAudienceSegment",
"id": "225702933721171456"
},
/* omitted if no errors */
"errors": [],
/* omitted if no warnings */
"warnings": []
}
Note that this will only wipe all of the users from the audience segment and will not delete the audience segment itself.
Note on Audience Computation
Audience updates are processed daily at 0h UTC and 12h UTC and can take around 5 hours to reflect on a live campaign. This is important for audiences that are frequently updated as changes should be ready for processing prior to these two times.
Updated about 1 month ago