Line Items Endpoints
Getting Started
- A line item holds promoted products to advertise on a single retailer.
- Line items include basic settings such as start and end dates, optional budget controls, and the associated retailer where ads are served.
- Budgets can also be managed at the campaign level.
- Several reports are available to track line item performance.
- Campaigns are limited to 10,000 non-archived line items.
- Line items are automatically archived 90 days after their end date.
Endpoints
Method | Endpoint | Description |
---|---|---|
GET | /accounts/{accountId}/line-items | Retrieve all line items associated with a specific account. |
GET | /line-items/{lineItemId} | Retrieve details of a specific line item by its ID. |
GET | /retail-media/line-items/{lineItemId}/keywords/recommended | Retrieves a collection of recommended keywords for a line item |
Line Item Attributes
Omitting the status field in line item update calls will result in a 400 error. Ensure that you include the status when updating line items to avoid validation issues.
Data Type: string
Accepted Value: int64
Write? No
Nullable? No
Description: Line Item ID
type Required
Data Type: enum
Accepted Value: auction
, preferred
Write? No
Nullable? No
Description: Campaign type
campaignId Required
Data Type: string
Accepted Value: int64
Write? No
Nullable? No
Description: Campaign ID
name Required
Data Type: string
Accepted Value: 255 char limit
Write? Yes
Nullable? No
Description: Line item name; must be unique within a campaign
targetRetailerId Required
Data Type: string
Accepted Value: int64
Write? No
Nullable? No
Description: ID of the retailer the line item serves on
startDate Required
Data Type: date
Accepted Value: YYYY-MM-DD
Write? Yes
Nullable? No
Description: Line item start date in the account timeZone
endDate Optional
Data Type: date
Accepted Value: YYYY-MM-DD
Write? Yes
Nullable? Yes
Description: Line item end date in the account timeZone
; serves indefinitely if omitted or set to null
.
A timestamp can be included as well if the line item is desired to end at a certain time of day
budget Optional
Data Type: number
Accepted Value: at least 0
Write? No
Nullable? Yes
Description: Line item lifetime spend cap; uncapped if omitted or set to null
budgetSpent
Data Type: number
Accepted Value: at least 0
Write? No
Nullable? No
Description: The amount the line item has already spent
budgetRemaining
Data Type: number
Accepted Value: 0 and budget
Write? No
Nullable? Yes
Description: Amount the line item has to remain until cap is hit; null if budget is uncapped
status
Data Type: enum
Accepted Value: active
, paused
, scheduled
, ended
, budgetHit
, noFunds
, draft
, archived
Write? Yes
Nullable? No
Description: Line item status; can only be updated by a user to active
or paused
; all other values are applied automatically depending on flight dates, financials, or missing attributes required for line item to serve.
To understand the conditions that will cause a status to change, check out our status page
createdAt Optional
Data Type: timestamp
Accepted Value: ISO-8601
Write? No
Nullable? No
Description: Timestamp in UTC of last line item update
Get All Line Items
This endpoint lists all line items in the specified campaign. Results are paginated.
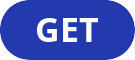
https://api.criteo.com/{version}/retail-media/accounts/{accountId}/line-items
Sample Request
curl -X GET "https://api.criteo.com/{version}/retail-media/accounts/123456/line-items" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
import requests
url = "https://api.criteo.com/{version}/retail-media/accounts/4/line-items"
payload={}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/accounts/4/line-items")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/accounts/4/line-items');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": [
{
"type": "RetailMediaLineItem",
"id": "9979917896105882144",
"attributes": {
"campaignId": "8343086999167541140",
"name": "Line Item 123",
"targetRetailerId": "3239117063738827231",
"startDate": "2020-04-06",
"endDate": null,
"budget": null,
"budgetSpent": 2383.87,
"budgetRemaining": null,
"status": "active",
"createdAt": "2020-04-06T17:29:11+00:00",
"updatedAt": "2020-04-06T17:29:11+00:00"
}
},
// ...
{
"type": "RetailMediaLineItem",
"id": "6854840188706902009",
"attributes": {
"campaignId": "8343086999167541140",
"name": "Line Item 789",
"targetRetailerId": "18159942378514859684",
"startDate": "2020-04-08",
"endDate": null,
"budget": 8000.00,
"budgetSpent": 1921.23,
"budgetRemaining": 6078.77,
"status": "paused",
"createdAt": "2020-04-06T23:42:47+00:00",
"updatedAt": "2020-06-03T03:01:52+00:00"
}
}
],
"metadata": {
"totalItemsAcrossAllPages": 105,
"currentPageSize": 25,
"currentPageIndex": 0,
"totalPages": 5,
"nextPage": "https://api.criteo.com/{version}/retail-media/accounts/123456/line-items?pageIndex=1&pageSize=25",
"previousPage": null
}
}
Get a Specific Line Item
This endpoint retrieves details for a specified line item by its ID
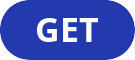
https://api.criteo.com/{version}/retail-media/line-items/{lineItemId}
Sample Request
curl -X GET "https://api.criteo.com/{version}/retail-media/line-items/2465695028166499188" \
-H "Authorization: Bearer <MY_ACCESS_TOKEN>"
import requests
url = "https://api.criteo.com/{version}/retail-media/line-items/2465695028166499188"
payload={}
headers = {
'Accept': 'application/json',
'Authorization': 'Bearer <MY_ACCESS_TOKEN>'
}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.criteo.com/{version}/retail-media/line-items/2465695028166499188")
.method("GET", body)
.addHeader("Accept", "application/json")
.addHeader("Authorization", "Bearer <MY_ACCESS_TOKEN>")
.build();
Response response = client.newCall(request).execute();
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.criteo.com/{version}/retail-media/line-items/2465695028166499188');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Accept' => 'application/json',
'Authorization' => 'Bearer <MY_ACCESS_TOKEN>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Sample Response
{
"data": {
"type": "RetailMediaLineItem",
"id": "2465695028166499188",
"attributes": {
"campaignId": "8343086999167541140",
"name": "My New Line Item",
"targetRetailerId": "18159942378514859684",
"startDate": "2020-04-06",
"endDate": null,
"budget": null,
"budgetSpent": 0.00,
"budgetRemaining": null,
"status": "draft",
"createdAt": "2020-04-06T06:11:23+00:00",
"updatedAt": "2020-04-06T06:11:23+00:00"
}
}
}
Responses
Response | Description |
---|---|
🟢 200 | Call completed successfully. The specified line item details are returned. |
🔴 403 | API user is not authorized to make requests for the account ID. To request authorization, follow the authorization request steps. |
🔴 404 | Line item ID not found. Ensure the lineItemId is correct and exists. |
Updated 9 days ago