Consent URL Generation
Overview
The Criteo API allows you to build custom apps that help the world's advertisers grow their businesses.
In order for your app to function, your users will have to delegate permissions for one or more of the Criteo advertisers that they oversee.
To do this, you will need to direct the user to a unique consent delegation page. The URLs for this type of page can be generated in one of two ways: manually from your Criteo App page, or programmatically using a cryptographic key.
Both methods are described below.
In this guide, we assume you have completed all the steps in the API application setup section, and you already have your application tokens.
Generate Consent URL
Client Credentials
For client credential application, once you have logged in to the Criteo Partners Portal, find your application under My apps
and navigate to your Application details
page.
At the top of your App
page, you will see a Generate new URL
button. Click the button to generate a single consent URL which can be easily copied to your clipboard.
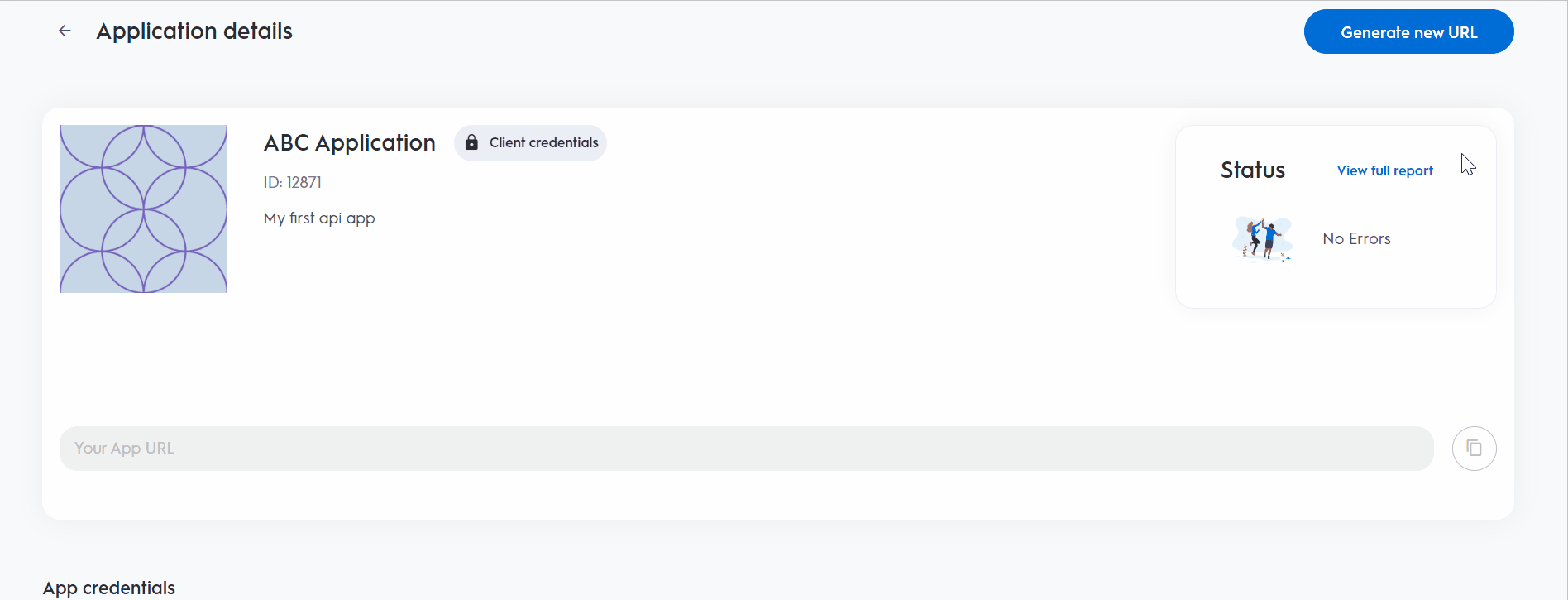
The consent URL has two query parameters:
https://consent.criteo.com/request?nonce=<nonce>&hmac=<signature>
Each button click will generate a new URL. A fresh consent URL must be generated whenever a new authorization is needed from the advertiser.
Consent URL Expiration
The consent URL expires 30 days after creation. It is valid for a single use, meaning once it has been used to grant consent, it cannot be reused for future authorizations.
Share the consent URL with an admin or business manager of the advertiser account you need access to. The user will be redirected to the Criteo's consent portal to approve your API application access.
For more details, consent granters should follow the instructions provided on the Authorization Request page.
Authorization Code
Generating a consent URL for authorization code applications requires a separate process. This option is not available under the Application details
page. For guidance on generating the consent URL, refer to the instructions for the Authorization Code application setup.
Generate Consent URLs Programmatically
To generate consent URLs dynamically, you need a public and private key pair for signing the URLs.
To obtain this key pair, you must register a callback URL to receive confirmations. Details on the callback process are covered in a later section.
Log in to the Criteo Partners Portal and navigate to your app page by selecting your app in the My application details
section.
Scroll to the Connector Parameters
section and click Create a new connector
. You will be prompted to enter a callback URL. After registration, the callback URL can be modified at any time, while the associated key pair remains the same.
Upon completion, your browser will download a .txt
file containing your public and private key pair.
Signing Keypair Generation
The private signing key is shared only once when you register a callback URL. Please ensure to store it securely. If you lose your private key, you can delete and re-register your callback URL to obtain a new one.
- With the signing key pair, you can programmatically generate a consent URL using the HMAC-SHA512 hashing algorithm to create a
signature
.
The consent URL has the following structure and parameters:
https://consent.criteo.com/request?key=<public signing key>×tamp=<UNIX timestamp in seconds>&state=<state>&redirect-uri=<redirect URI>&signature=<hashed signature>
Parameter | Description |
---|---|
key | Your public signing key |
timestamp | The UNIX timestamp of when your URL was generated, in seconds |
state | An arbitrary string to be included in the consent callback (e.g., the User ID of your app user) |
redirect-uri | The URL to redirect the user to after consent delegation |
signature | The HMAC-SHA512 hashed query string of the previous four parameters, in order |
The signature
is created by hashing the following string using the key
, timestamp
, state
, and redirect-uri
values:
?key=<public signing key>×tamp=<UNIX timestamp in seconds>&state=<state>&redirect-uri=<redirect URI>
Code examples for generating a Consent URL can be found below:
const sha512 = require('js-sha512').sha512;
function generateConsentURL(publicSigningKey, signingSecret, redirect, state){
const timestamp = Math.round(Date.now() / 1000);
const query = `?key=${publicSigningKey}×tamp=${timestamp}&state=${state}&redirect-uri=${redirect}`;
return `https://consent.criteo.com/request${query}&signature=${sha512.hmac(signingSecret, query)}`;
}
const res = generateConsentURL('public key', 'private key', 'https://example.com/app-landing-page', 'userID');
console.log(res);
using System;
using System.Text;
using System.Security.Cryptography;
/*
Enter your public signing key and signing secret in between the quotations below. You can also choose to modify your redirect URL (where your consent giver will be redirected too after they accept your consent request). You can also choose to pass in parameters inside the state object.
*/
public class Program
{
public static void Main()
{
// MODIFY THIS
var publicSigningKey = "<public signing key>";
var signingSecret = "<private signing key>";
var redirectUri = "https://developers.criteo.com/";
var state = "userID";
//
long timestamp = ((DateTimeOffset)DateTime.Now).ToUnixTimeSeconds();
var signature = createSignature(publicSigningKey, timestamp, state, redirectUri, signingSecret);
Console.WriteLine(generateUrl(publicSigningKey, timestamp, state, redirectUri, signature));
}
public static string createSignature(string publicSigningKey, long timestamp, string state, string redirectUri, string signingSecret)
{
var message = $"?key={publicSigningKey}×tamp={timestamp}&state={state}&redirect-uri={redirectUri}";
return HmacUtil.Sign(message, Encoding.UTF8.GetBytes(signingSecret));
}
public static string generateUrl(string publicSigningKey, long timestamp, string state, string redirectUri, string signature)
{
return $"https://consent.criteo.com/request?key={publicSigningKey}×tamp={timestamp}&state={state}&redirect-uri={redirectUri}&signature={signature}";
}
}
public static class HmacUtil
{
public static string Sign(string message, byte[] signingKeyBytes)
{
using (var hmac = new HMACSHA512(signingKeyBytes))
{
var hashedMessage = hmac.ComputeHash(Encoding.UTF8.GetBytes(message));
var stringBuilder = new StringBuilder();
foreach (var value in hashedMessage)
{
stringBuilder.Append(value.ToString("x2"));
}
return stringBuilder.ToString();
}
}
}
Consent Delegation Callback
After the user completes the Consent Delegation flow, an HTTP callback will be sent to the registered URL. The POST
body will include a Type
field, indicating whether the consent was successful (ConsentGranted
) or unsuccessful (ConsentDenied
). For a ConsentDenied
callback, AcceptedScopes
will be an empty array.
Callback Body Update
CriteoService
values have been updated for consistency. Possible values areMarketingSolutions
andRetailMedia
.
{
"Type": "ConsentGranted",
"Data": {
"Key": "971062d8161ba4ef8f78f3201a6f361f",
"Timestamp": 1614366053,
"State": "",
"ApplicationId": 2,
"ApplicationName": "Test App",
"RequestedScopes": [
{
"AccessLevel": "Read",
"Domain": "Analytics",
"CriteoService": "MarketingSolutions"
}
],
"AcceptedScopes": [
{
"AccessLevel": "Read",
"Domain": "Analytics",
"CriteoService": "MarketingSolutions"
}
],
"Advertisers": [
{
"Id": "12345",
"Name": "Example Advertiser"
}
]
}
}
For Marketing Solutions apps, shared entities are listed under Advertisers
, while for Retail Media apps, they are under Accounts
.
The callback request includes an HTTP header named x-criteo-hmac-sha512
, which contains the HMAC-SHA512
hash of the callback request body. Use your app's signing secret to validate the request's integrity and authenticity.
Callbacks only for programmatically generated URLs
Callbacks are sent only for dynamically generated consent URLs. You will not receive a
POST
when user consent is provided through a link generated directly from the Developer Dashboard..
User Redirection
Once the Consent Delegation is complete, the POST
request to the callback URL and user redirection to the redirect URI occur in sequence. The user is not redirected until the callback attempt resolves.
If the first callback attempt fails, it is retried up to two more times before redirecting the user. After three failed attempts, the user is redirected, and Criteo logs the callback error.
Updated 3 months ago