Consent URL Generation
Overview
The Criteo API allows you to build custom apps that help the world's advertisers grow their businesses.
In order for your app to function, your users will have to delegate permissions for one or more of the Criteo advertisers that they oversee. To do this, you will need to direct the user to a unique consent delegation page. The URLs for this type of page can be generated in one of two ways - manually from your Criteo App page, or programmatically using a cryptographic key.
Both methods are described below
In this guide we assume you have completed all the steps in the API application setup section, and you already have your application tokens.
Generate Consent URL
Client Credentials
For client credential application, once you have logged in to the Criteo Partners Portal, find your application under the "My apps" and navigate to your "Application details" page.
At the top of your App page, you will see a Generate new URL button. Click the button to generate a single consent URL which can be easily copied to your clipboard
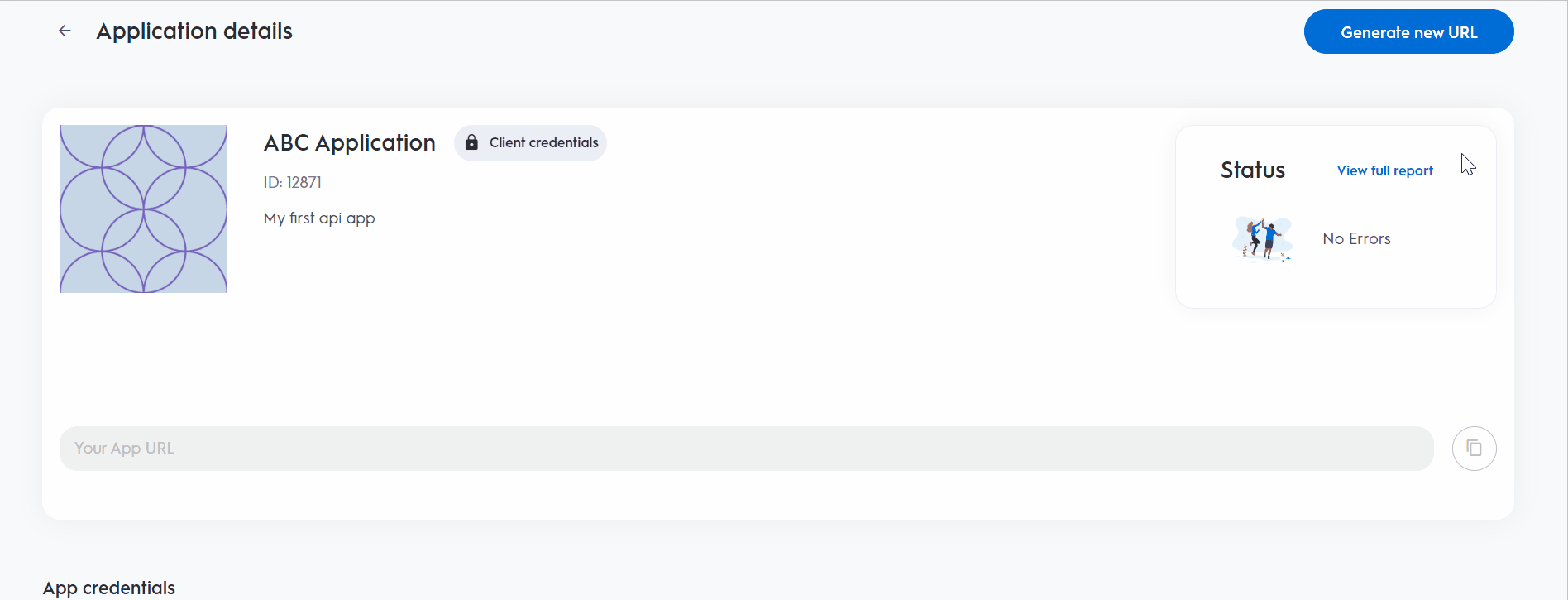
The URL will have two query parameters as shown below:
https://consent.criteo.com/request?nonce=<nonce>&hmac=<signature>
Each click of the button will generate a new URL. You will need to generate a new consent URL each time a new authorization is necessary from the advertiser.
Consent URL Expiration
The consent URL will expire after 30 days. Each consent URL is only valid for a single use, and once it has been used to grant consent, it will no longer work for future authorizations.
Share the consent URL with an admin, business manager or technical manager of the advertiser account you will need access. The user will be redirected to the Criteo consent portal to approve your API application access.
- For more details consent granters will need to follow the instructions provided in the Authorization Request page
Authorization Code
Authorization code applications requires a separate consent URL generation. You will notice this option is not available under the "Application details" page. For instructions of how to generate the consent URL, make sure to follow the instructions for the Authorization Code application setup.
Generate Consent URLs Programmatically
In order to generate consent URLs on the fly, you will need a public and private keypair for signing your URLs.
To obtain this keypair, you will need to register a callback URL to receive confirmations. The callback process is described in full in a later section.
Once you have logged in to the Criteo Partners Portal, navigate to your app page by clicking your app's card in the My application details section.
Scroll down to the Connector Parameters section and click Create a new connector. You will be prompted to enter a callback URL. Once registered, your callback URL can be modified at any time and the keypair associated will remain the same.
Once complete, your browser will download a .txt
file with your public and private keypair.
Signing Keypair Generation
The private signing key is only shared once at the time you register a callback URL. Keep it somewhere safe. If you lose your private key, you can delete and re-register your callback URL to obtain a new one.
- Once you have your signing keypair, you will be able to programmatically generate a consent URL.
- Doing so requires that you use a HMAC-SHA512 hashing algorithm to generate a
signature
.
The consent URL will have the following structure and parameters:
https://consent.criteo.com/request?key=<public signing key>×tamp=<UNIX timestamp in seconds>&state=<state>&redirect-uri=<redirect URI>&signature=<hashed signature>
Parameter | Description |
---|---|
key | Your public signing key |
timestamp | The UNIX timestamp of when your URL was generated, in seconds |
state | An arbitrary string to be included in the consent callback. For example, the User ID of your app user. |
redirect-uri | The URL to redirect the user to after consent delegation |
signature | The HMAC-SHA512 hashed query string of the previous four parameters, in order |
The final parameter, the signature
, should be the HMAC-SHA512 hashed value of the following string, using the same key
, timestamp
, state
and redirect-uri
values as your URL:
?key=<public signing key>×tamp=<UNIX timestamp in seconds>&state=<state>&redirect-uri=<redirect URI>
Code examples for generating a Consent URL can be found below:
const sha512 = require('js-sha512').sha512;
function generateConsentURL(publicSigningKey, signingSecret, redirect, state){
const timestamp = Math.round(Date.now() / 1000);
const query = `?key=${publicSigningKey}×tamp=${timestamp}&state=${state}&redirect-uri=${redirect}`;
return `https://consent.criteo.com/request${query}&signature=${sha512.hmac(signingSecret, query)}`;
}
const res = generateConsentURL('public key', 'private key', 'https://example.com/app-landing-page', 'userID');
console.log(res);
using System;
using System.Text;
using System.Security.Cryptography;
/*
Enter your public signing key and signing secret in between the quotations below. You can also choose to modify your redirect URL (where your consent giver will be redirected too after they accept your consent request). You can also choose to pass in parameters inside the state object.
*/
public class Program
{
public static void Main()
{
// MODIFY THIS
var publicSigningKey = "<public signing key>";
var signingSecret = "<private signing key>";
var redirectUri = "https://developers.criteo.com/";
var state = "userID";
//
long timestamp = ((DateTimeOffset)DateTime.Now).ToUnixTimeSeconds();
var signature = createSignature(publicSigningKey, timestamp, state, redirectUri, signingSecret);
Console.WriteLine(generateUrl(publicSigningKey, timestamp, state, redirectUri, signature));
}
public static string createSignature(string publicSigningKey, long timestamp, string state, string redirectUri, string signingSecret)
{
var message = $"?key={publicSigningKey}×tamp={timestamp}&state={state}&redirect-uri={redirectUri}";
return HmacUtil.Sign(message, Encoding.UTF8.GetBytes(signingSecret));
}
public static string generateUrl(string publicSigningKey, long timestamp, string state, string redirectUri, string signature)
{
return $"https://consent.criteo.com/request?key={publicSigningKey}×tamp={timestamp}&state={state}&redirect-uri={redirectUri}&signature={signature}";
}
}
public static class HmacUtil
{
public static string Sign(string message, byte[] signingKeyBytes)
{
using (var hmac = new HMACSHA512(signingKeyBytes))
{
var hashedMessage = hmac.ComputeHash(Encoding.UTF8.GetBytes(message));
var stringBuilder = new StringBuilder();
foreach (var value in hashedMessage)
{
stringBuilder.Append(value.ToString("x2"));
}
return stringBuilder.ToString();
}
}
}
Consent Delegation Callback
Once the user has completed the Consent Delegation flow, an HTTP callback will be sent to the URL associated with the signing keypair you used.The POST
body will include a Type
field, which will indicate either a successful ( value of ConsentGranted
) or unsuccessful (value of ConsentDenied
) consent request.
For a ConsentDenied
callback, AcceptedScopes
will be an empty array.
Callback Body Update
CriteoService
values have been updated to improve consistency. Possible values areMarketingSolutions
andRetailMedia
{
"Type": "ConsentGranted",
"Data": {
"Key": "971062d8161ba4ef8f78f3201a6f361f",
"Timestamp": 1614366053,
"State": "",
"ApplicationId": 2,
"ApplicationName": "Test App",
"RequestedScopes": [
{
"AccessLevel": "Read",
"Domain": "Analytics",
"CriteoService": "MarketingSolutions"
}
],
"AcceptedScopes": [
{
"AccessLevel": "Read",
"Domain": "Analytics",
"CriteoService": "MarketingSolutions"
}
],
"Advertisers": [
{
"Id": "12345",
"Name": "Example Advertiser"
}
]
}
}
Other fields include the public Key
, Timestamp
, and State
values provided in the original consent URL.
Please note that for Marketing Solutions apps, the entities that have been shared will be in the "Advertisers" field of the callback body, but for Retail Media apps, those entities will be in an "Accounts" field.
The callback request will also include an HTTP header named x-criteo-hmac-sha512
. Its value will be the HMAC-SHA512 hash of the callback request body, using the same signing secret value as your app.
In this way, you can use your app's signing secret to validate the integrity and authenticity of the callback.
Callbacks only for programmatically generated URLs
Callbacks will only be sent for dynamically generated consent URLs. You will not receive a
POST
to your callback URL when user consent is provided using a link generated directly from Developer Dashboard.
User Redirection
Once the user has completed the Consent Delegation flow, the POST
request to the callback URL and the user's redirection to the redirect URI are executed in sequence. The user will not be redirected until the callback attempt has resolved.
If the call to the callback URL fails on its first attempt, the POST
will be retried up to two more times before the user is redirected to the redirect URL.
Following three failed attempts, the user will be redirected to the redirect URL and Criteo will log the callback error.
Updated 14 days ago