BeaconSDK
Automatically track beacons by sending the beacon URLs to the HTML DOM.
Introduction
The BeaconSDK
library is designed to simplify ad tracking for developers.
When a retailer integrates with Criteo’s Retail Media ecosystem using the Direct Ad Server solution, the event-tracking logic needs to be implemented by the retailer’s own development team.
This task can be time-consuming, difficult to review, and generate non-standardized data. BeaconSDK
solves that by providing industry-compliant viewability measurement, using the same viewability facilities from Criteo’s OneTag solutions.
BeaconSDK
supports all of Criteo’s standard and proprietary ad formats and provides both placement and product-level tracking.
Process overview integrating with the BeaconSDK
For more information on the different levels of beacons, please check the ad tracking introduction page.
With BeaconSDK integrated into your project, the ad rendering and beaconing phase should be considerably shortened.
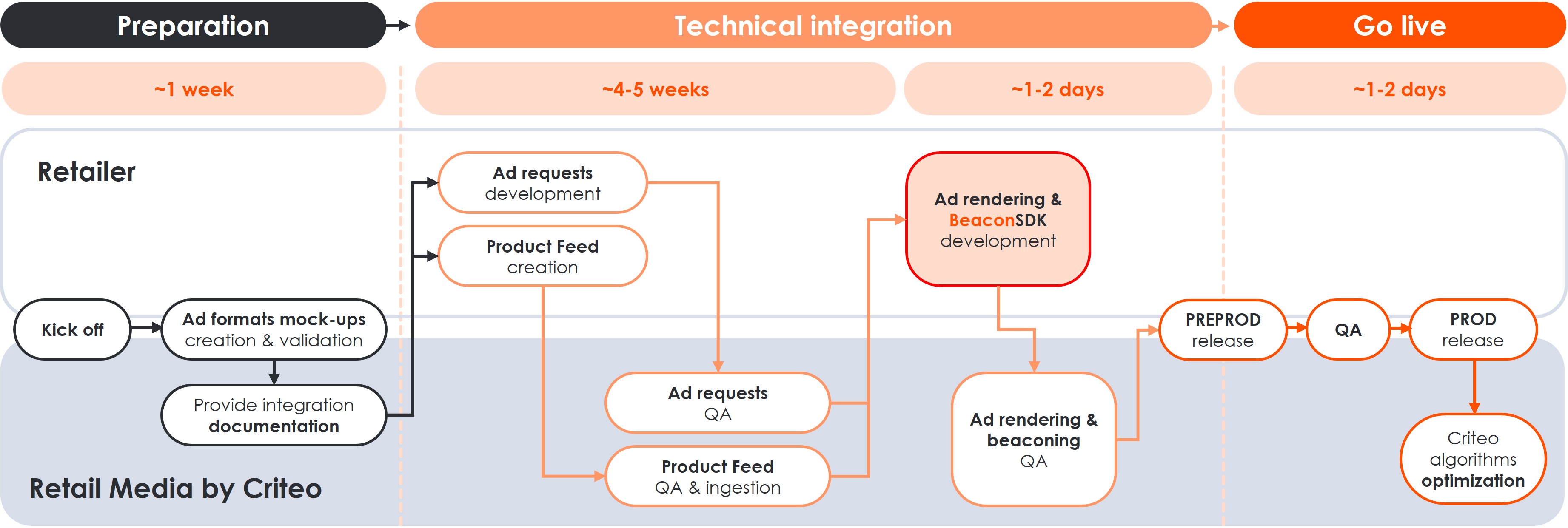
Quick Start
Criteo’s Delivery API returns unique beacon URLs for each placement and product (SKU). To start tracking with BeaconSDK using the new static JavaScript bundle, follow these steps:
Add Beacon Attributes to your HTML
Each beacon URL returned by the Delivery API response must be attached to the corresponding HTML element using a data-
attribute in the format data-criteo-[beacon-level]-[beacon-type]
<div class="sponsored-product-placement"
data-criteo-placement-onloadbeacon="//b.criteo.com/rm?rm_e=4cp0JZowKy"
data-criteo-placement-onviewbeacon="//b.criteo.com/rm?rm_e=rM9TNBqBpz"
data-criteo-placement-onclickbeacon=""
>
<div class="sponsored-product-tile"
data-criteo-product-onloadbeacon="//b.criteo.com/rm?rm_e=q5CjxkuM0B"
data-criteo-product-onviewbeacon="//b.criteo.com/rm?rm_e=04im9RY12L"
data-criteo-product-onclickbeacon="//b.criteo.com/rm?rm_e=9dUq1vC2Ns"
>
<!--- Product Content -->
</div>
<!-- Placement Content -->
</div>
[beacon-level]
is one ofproduct
orplacement
, and[beacon-type]
is one ofonloadbeacon
,onviewbeacon
oronclickbeacon
.
Load BeaconSDK
At the end of your HTML, include the BeaconSDK bundle using the script tag:
<script src="https://static.criteo.net/cbs/prod/v3/BeaconSDK/bundle.js" data-dynamic-tracking="true" async></script>
Example
Here's a simple example that uses only sponsored products and BeaconSDK to track OnLoad
, OnView
, and OnClick
events. We also added a simple JavaScript function to handle add-to-cart (OnBasketChange
) and add-to-wishlist (OnWishlist
) beacons.
Usage
In its most basic form, BeaconSDK will automatically observe and track all HTML elements that include attributes matching the format data-criteo-[beacon-level]-[beacon-type]
BeaconSDK supports the following beacon types:
OnLoadBeacon
— triggered as soon as the SDK detects the HTML element, regardless of its visibility in the viewport.OnViewBeacon
— triggered when the element meets the IAB’s Viewable Impression Guidelines.OnClickBeacon
— triggered by default when the corresponding HTML element is clicked.
All beacon URLs are sent using the navigator.sendBeacon()
method.
Dynamic Tracking
By default, BeaconSDK will only track HTML elements that are present in the DOM at the time the script is loaded.
To enable dynamic tracking of elements added later, use the data-dynamic-tracking="true"
parameter on the script tag:
<script src="https://static.criteo.net/cbs/prod/v3/BeaconSDK/bundle.js" data-dynamic-tracking="true" async></script>
When this parameter is enabled, BeaconSDK uses the [MutationObserver API](https://developer.mozilla.org/en-US/docs/Web/API/MutationObserver) to monitor changes in the DOM and register new elements that match the data-criteo-*
pattern.
Restricting Tracking to a Specific Container
By default, the SDK observes all subnodes of the <body>
element. If you want to restrict the tracking to a specific container or a shadow DOM root, you can specify a container using the data-container-selector
attribute:
<script src="https://static.criteo.net/cbs/prod/v3/BeaconSDK/bundle.js"
type="text/javascript"
data-dynamic-tracking="true"
data-container-selector=".sponsored-products"
async></script>
This limits DOM observation to elements within the specified container selector.
Debugging
To help debug beacon tracking in your browser, you can enable debug logs by setting the following cookie:
criteo-viewability-debug=true
Once enabled, BeaconSDK will log each beacon it sends to the browser console. These logs start with the prefix Criteo: sent [beacon-level]-[beacon-type] beacon:
.
You can filter your browser console by the keyword Criteo
to isolate these logs.
Each log entry includes an object with the following properties:
BeaconType
: a string indicating the type of beacon sentBeaconURL
: the URL that was called viasendBeacon()
HTMLElement
: the actual DOM element that triggered the beacon (hovering it in DevTools will highlight the element)
Example
Criteo: sent product-onLoad beacon:
{
BeaconType: "product-onLoad",
BeaconURL: "//b.us.criteo.com/rm?rm_e=productTwoOnLoadBeacon",
HTMLElement: <div class="sponsored-product-tile hoverable waves-effect">...</div>
}
Getting BeaconSDK
We offer three options to get started with BeaconSDK.
Static Script Tag (Recommended)
You can directly include the BeaconSDK by adding the following <script>
tag to your website:
<script src="https://static.criteo.net/cbs/prod/v3/BeaconSDK/bundle.js" async></script>
You should place this script tag at the end of your <body>
tag, just before the closing </body>
tag. This ensures that the SDK loads after your page content. If your website dynamically loads content, see the section on Dynamic Tracking
OneTag Bundle
If you already use Criteo’s OneTag for Marketing Solutions or offsite campaigns, BeaconSDK can be automatically included via the OneTag bundle.
Please consult your Technical Account Manager (TAM) to confirm whether your OneTag setup supports BeaconSDK and to enable it if needed.
TypeScript NPM Package
If you prefer to integrate BeaconSDK directly into your build process, you can request the latest version of the npm package from your Technical Account Manager (TAM).
This option is only recommended if you want to build the SDK as part of your project and serve it from your own infrastructure.
See installation instructions below.
Installing and Self-hosting
BeaconSDK can be provided as a compressed TypeScript package. Once transpiled, the library is compliant with both CommonJS and ES6+ environments.
If you want to install and run BeaconSDK locally, ask your Technical Account Manager (TAM) for the package.
Installation
- Run
npm install
to ensure that TypeScript is installed in your project. - Download the
.tgz
file received from your TAM. It will be named in the format:criteo-beacon-sdk-x.y.z.tgz
(wherex.y.z
is the SDK version). - Move the
.tgz
file into your project directory. - Run:
Make sure to replace
1. npm install criteo-beacon-sdk-x.y.z.tgz
x.y.z
with the actual version string.
After the installation, you can import Import the necessary classes from the SDK into your JavaScript file:
import PlacementLevelBeaconHandler from '/beacon-sdk/PlacementLevelBeaconHandler.js';
import ProductLevelBeaconHandler from '/beacon-sdk/ProductLevelBeaconHandler.js';
import ViewabilityVisualizer from '[PROJECT_DIRECTORY]/debug/SkuViewabilityVisualizer'; // debug porposes only
// Attach handlers to the global window object for easy access
window.PlacementLevelBeaconHandler = PlacementLevelBeaconHandler;
window.ProductLevelBeaconHandler = ProductLevelBeaconHandler;
Use the provided functions
You can then start using the functions provided by BeaconSDK:
/* [...]
PlacementLevelBeaconHandler.attachOnViewBeacons();
PlacementLevelBeaconHandler.triggerOnLoadBeacons();
/* [...]
Placement-level beacon handler
The PlacementLevelBeaconHandler
class provides various methods for handling placement-level beacons. These beacons track user interactions with different ad placements.
triggerOnLoadBeacons()
: sendsonLoad
beacons for elements with thedata-criteo-placement-onloadbeacon
attribute. This method iterates over all elements with the specified attribute and sends a beacon for each element when the page loads.attachOnViewBeacons()
: attaches beacons to placements with thedata-criteo-placement-onviewbeacon
attribute. This method uses the IntersectionObserver API to monitor the visibility of ad placements and sends a beacon when an ad placement meets the viewability threshold according to IAB’s Viewable Impression Guidelines.attachOnClickBeacons([jsEvent])
: attachesonClick
beacons to placements with thedata-criteo-placement-onclickbeacon
attribute. If a JavaScript event is not provided, the default'click'
event will be used.triggerOnClickBeacon(beaconUrl, element)
: sendsonClick
beacons for placement-level elements. You should use this method if you want to handle the click beaconing together with other routines. It should be called with the beacon URL when a user clicks on an ad placement. An optional element parameter can be used to associate the beacon with a specific element.triggerOnFileClickBeacon(beaconUrl, element)
: sends onFileClick beacons for placement-level elements. This method should be called with the beacon URL when a user clicks on a file within an ad placement. An optional element parameter can be used to associate the beacon with a specific element.triggerOnBundleBasketChangeBeacon(beaconUrl, element)
: sends onBundleBasketChange beacons for placement-level elements. This method should be called with the beacon URL when there is a change in the bundle basket within an ad placement. An optional element parameter can be used to associate the beacon with a specific element.
Product-level beacon handler
The ProductLevelBeaconHandler
class provides various methods for handling product-level beacons. These beacons track user interactions with individual products (SKUs) within an ad unit.
triggerOnLoadBeacons()
: sendsonLoad
beacons for elements with thedata-criteo-product-onloadbeacon
attribute. This method iterates over all elements with the specified attribute and sends a beacon for each element when the page loads.attachOnViewBeacons()
: attaches beacons to products with thedata-criteo-product-onviewbeacon
attribute. This method uses the IntersectionObserver API to monitor the visibility of ad placements and sends a beacon when an ad placement meets the viewability threshold according to IAB’s Viewable Impression Guidelines.attachOnClickBeacons([jsEvent])
: attachesonClick
beacons to placements with thedata-criteo-product-onclickbeacon
attribute. If a JavaScript event is not provided, the click event will be used by default. The function will only attach to products loaded to the DOM with the specified attribute.triggerOnBasketChangeBeacon(beaconUrl, element)
Tracks when a user adds or removes an item from their shopping cart. An optionalelement
parameter can be used to associate the beacon with a specific element.triggerOnClickBeacon(beaconUrl, element)
Tracks when a user clicks on a product within an ad unit. An optionalelement
parameter can be used to associate the beacon with a specific element. You should use this method if you want to handle the click beaconing together with other routines.
Viewability visualizer
Once a product's view beacon is sent, the product viewability visualizer displays a checkmark on the product tile for 3 seconds and then restores the element to its original state and style.
This makes it easier for developers to visually check the corresponding view beacons that have just been sent.
To enable the viewability visualizer, import the visualizer and call the observe method. This only needs to be called once to apply to all pages.
import { ViewabilityVisualizer } from '@criteo/beacon-sdk';
ViewabilityVisualizer.observe();
Please use the viewability visualizer only in pre-production environments since, if deployed, end users will see the checkmark animation.
Updated 2 months ago